Jan 9th, 2023
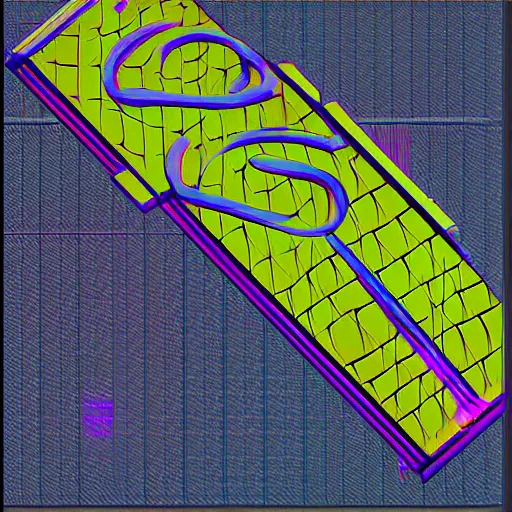
Welcome back to our Python3 intro course! In the last lesson, we learned about working with iteration and conditional statements in Python. These are important concepts to understand as they allow us to create more complex programs that can make decisions and perform actions based on certain conditions. In this lesson, we'll be exploring and learning how to define and call functions in Python, as well as how to work with input parameters and return values. By the end of this lesson, you should have a solid understanding of how to use functions in Python.
Functions are a way to group together a set of related instructions and give them a name, which can then be called upon when needed.
Functions can also accept input parameters, which allows us to customize their behavior and make them more versatile.
Functions are a cornerstone of programming and they allow you to break up your code into smaller, more manageable chunks.
This makes it easier to write, read, and understand your code, and it also makes it easier to reuse your code in different parts of your program.
Functions are defined using the def
keyword in Python, followed by the function name and a set of parentheses.
Inside the parentheses, you can specify any parameters or arguments that the function needs in order to run.
For example, you might define a function like this:
def greet(): print("Hello, World!")
This creates a function called greet
that simply prints "Hello, World!".
You can call a function by simply typing its name followed by a set of parentheses.
For example:
greet() # Output: Hello, World!
You can also pass arguments to a function, which are values that the function can use to perform its task. For example:
def greet(name): print("Hello, " + name + "!") greet("Alice") # Output: Hello, Alice! greet("Bob") # Output: Hello, Bob!
In this example, the greet
function takes a single argument, name
, and prints a personalized greeting using the value of name
.
You can also define default values for function arguments, which will be used if no value is provided when the function is called. For example:
def greet(name="World"): print("Hello, " + name + "!") greet() # Output: Hello, World! greet("Alice") # Output: Hello, Alice! greet("Bob") # Output: Hello, Bob!
In this example, the default value of name
is "World", so if no value is provided when the function is called, it will use this default value.
Functions can also return values using the return
keyword. For example:
def add(x, y): return x + y result = add(5, 6) # result is now 11
In this example, the add function takes two arguments, x
and y
, and returns their sum.
I hope this has been a helpful introduction to using functions in Python. Functions are an essential tool for organizing and simplifying your code, so be sure to practice and get comfortable with them. In the next lesson, we'll be exploring how to work with modules and libraries in Python. Stay tuned!