Jan 8th, 2023
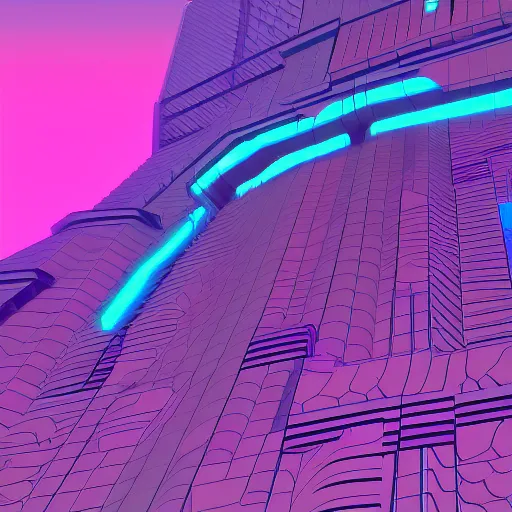
Welcome back to our Python3 intro course! In the last lesson, we learned about variables and data types in Python. These are fundamental concepts in programming that allow us to store and manipulate data in our programs. In this lesson, we'll be looking at more advanced concepts, including lists, loops and conditional statements. These are powerful tools that allow us to perform complex operations on our data and make our programs more dynamic.
Lists are an essential data structure in Python, allowing you to store and organize data in an ordered sequence. You can create a list by enclosing a comma-separated sequence of values in square brackets. Lists can contain elements of any data type, including other lists, making them a very flexible data structure. You can access the elements of a list using their index, which is the position of the element in the list. You can also modify the elements of a list by assigning new values to them using their index. Lists also have a number of built-in methods that allow you to perform various operations on them, such as adding or removing elements, sorting the list, and more. We'll be learning more about lists and how to work with them in Python in this lesson. For example:
fruits = ['apple', 'banana', 'mango']
This creates a list of three fruit strings. We'll use them for iteration more in this blog post, but will cover them more in depth in our post about data structures.
Loops allow you to execute a block of code multiple times, either a set number of times or until a certain condition is met.
There are two types of loops in Python: for
loops and while
loops.
A for
loop is used to iterate over a sequence, such as a list or a string. For example:
fruits = ['apple', 'banana', 'mango'] for fruit in fruits: print(fruit)
This will print each fruit in the fruits
list on a separate line.
A while
loop, on the other hand, will execute a block of code as long as a certain condition is True
.
For example:
i = 1 while i < 6: print(i) i += 1
This will print the numbers 1 through 5 on separate lines.
Conditional statements, also known as if
statements, allow you to execute a block of code only if a certain condition is met.
For example:
x = 10 if x > 5: print("x is greater than 5")
This will print "x is greater than 5" because the condition x > 5
is True
.
You can also use elif
statements to check for multiple conditions, and an else
statement to specify a block of code to be executed if none of the conditions are met.
For example:
x = 10 if x > 15: print("x is greater than 15") elif x > 5: print("x is greater than 5 but less than or equal to 15") else: print("x is less than or equal to 5")
This will print "x is greater than 5 but less than or equal to 15" because x
is greater than 5 but not greater than 15.
I hope this has been a helpful introduction to loops and conditional statements in Python. These concepts will be essential as you continue to learn and work with Python, so be sure to practice and get comfortable with them. In the next lesson, we'll be looking at functions, which allow you to organize and reuse your code. Stay tuned!