Jan 7th, 2023
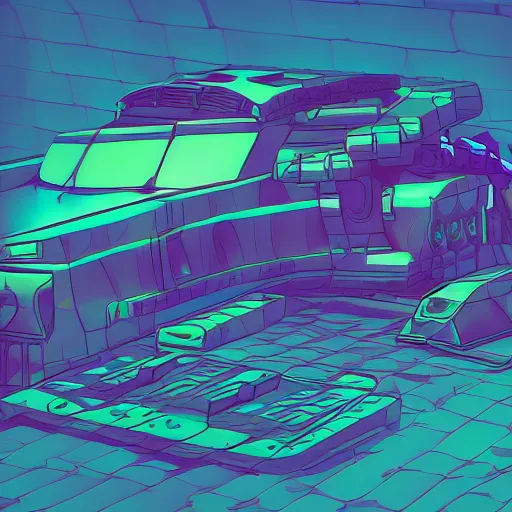
Welcome back to our Python3 intro course! In the last lesson, we covered how to set up your Python environment and create your first Python program. This is an important step in getting started with Python, as it helps you to understand how to run and debug your programs. In this lesson, we'll delve deeper into using variables and data types in Python.
Understanding variables and data types is a fundamental concept in programming, as it allows us to store and manipulate data in our programs. What you learn in this lesson will teach you the basics of how Python stores data, and these topics carry over to many other programming languages. Most languages include similar data types, such as integers, strings, characters, floating-point values and booleans. Each data type has its own characteristics and uses, and it's important to choose the right data type for the task at hand.
A variable is a named container for storing data.
In Python, you can create a variable by simply assigning a value to it using the assignment operator =
.
This operator is used to give a value to a variable, and it consists of the equal sign (=) with the value on the right side and the variable name on the left side.
For example:
x = 5 y = "hello"
Here, we've created two variables: x
, which contains the integer value 5, and y
, which contains the string "hello".
Python has several built-in data types, including integers, floating-point numbers, strings, and Booleans.
An integer is a whole number, either positive or negative. For example:
x = 5 y = -10
A floating-point number is a number with a decimal point. For example:
x = 3.14 y = -2.718
A string is a sequence of characters, either letters, numbers, or symbols, enclosed in either single or double quotation marks. For example:
x = "hello" y = '12345'
A Boolean is a data type that can have only two values: True
or False
.
For example:
x = True y = False
You can also use the type()
function to check the data type of a variable.
For example:
x = 5 print(type(x)) # Output: <class 'int'> y = "hello" print(type(y)) # Output: <class 'str'>
It's important to use the appropriate data type for your variables, as this can affect how your program behaves. For example, if you try to perform arithmetic operations on a string, you'll get an error.
x = "hello" y = 5 print(x + y) # Output: TypeError: can only concatenate str (not "int") to str
To avoid this, you can use type conversion functions to convert variables to the desired data type. For example:
x = "5" y = int(x) # y is now the integer 5 x = 5 y = str(x) # y is now the string "5"
I hope this has been a helpful introduction to using variables and data types in Python. In the next lesson, we'll look at more advanced concepts such as loops and conditional statements. Stay tuned!