Jan 13th, 2023
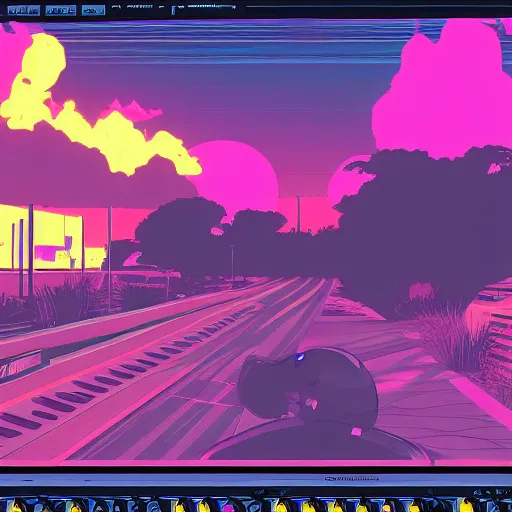
Hello and welcome back to my intro to Python series! In the previous post, we learned about exception handling and object-oriented programming in Python. These are powerful tools that allow you to write more organized and reusable code, and they are an important part of any Python programmer's toolkit. In this post, we're going to cover two more advanced OOP concepts: inheritance and polymorphism.
Inheritance is a way to create a new class that is a modified version of an existing class. The new class is called the subclass, and the existing class is the superclass. The subclass inherits attributes and behavior from the superclass, and can also have additional attributes and behavior of its own. This allows you to create a hierarchy of classes, where a subclass can inherit attributes and behavior from its superclass, and then further customize or override those attributes and behavior as needed. This is a powerful way to reuse code and avoid redundancy, as you can create a base class with common attributes and behavior, and then create subclasses that inherit from that base class and specialize it for specific purposes. For example:
class Animal: def __init__(self, name, species): self.name = name self.species = species def make_sound(self): print("Some generic animal sound") class Cat(Animal): def __init__(self, name, breed, toy): super().__init__(name, species="Cat") # call superclass's __init__ method self.breed = breed self.toy = toy def play(self): print(f"{self.name} plays with {self.toy}") cat1 = Cat("Kitty", "Siamese", "String") print(cat1.name) # prints "Kitty" print(cat1.species) # prints "Cat" cat1.make_sound() # prints "Some generic animal sound" cat1.play() # prints "Kitty plays with String"
In this example, we create a Cat
subclass that inherits from the Cat
class has its own __init__
method and a play
method, but it also has access to the attributes and make_sound
method of the Animal
class.
Polymorphism is the ability of a subclass to override or extend the behavior of its superclass. For example:
class Animal: def __init__(self, name, species): self.name = name self.species = species def make_sound(self): print("Some generic animal sound") class Cat(Animal): def __init__(self, name, breed, toy): super().__init__(name, species="Cat") self.breed = breed self.toy = toy def make_sound(self): print("Meow") class Dog(Animal): def __init__(self, name, breed): super().__init__(name, species="Dog") self.breed = breed def make_sound(self): print("Woof") animals = [Cat("Kitty", "Siamese", "String"), Dog("Fido", "Labrador")] for animal in animals: animal.make_sound()
In this example, we have a Cat
and a Dog
class that both inherit from the Animal
class.
However, each subclass has its own implementation of the make_sound
method, which overrides the one in the superclass.
When we call the make_sound
method on each animal in the list, it calls the correct method for each type of animal.
This is an example of polymorphism: the same method (make_sound
) behaves differently for different objects (Cat
and Dog
).
Inheritance and polymorphism are powerful tools in object-oriented programming that allow you to create flexible and reusable code. They can make your code more organized and easier to maintain.
I hope this post has helped you understand inheritance and polymorphism in Python. In the next post, we'll look at some more advanced topics in Python, such as modules and packages. Thanks for reading!