Jan 14th, 2023
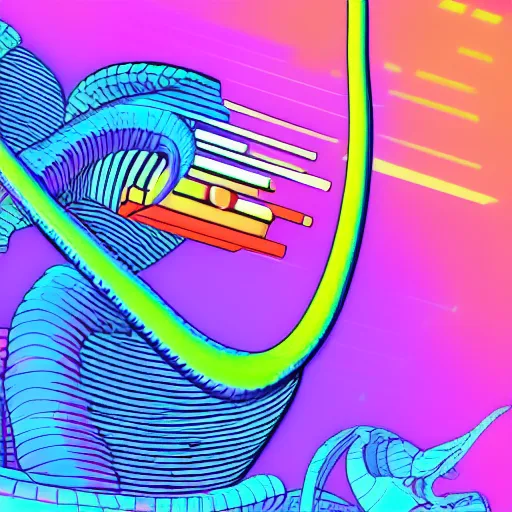
Hello and welcome back to my intro to Python series!
In the previous posts, we learned about advanced concepts such as exception handling, object-oriented programming, inheritance, and polymorphism.
These are powerful tools that allow you to write more organized and reusable code, and they are an important part of any Python programmer's toolkit.
In this post, we're going to look at two popular libraries for web scraping and parsing: requests
and Beautiful Soup
.
Web scraping is a technique for extracting data from websites, and it is a useful tool for a wide range of tasks, such as data mining, data analytics, and even automating tasks. In Python, you can use the requests library to make HTTP requests to websites and retrieve the HTML or other data that they return. The Beautiful Soup library is a popular library for parsing and navigating HTML and XML data, which makes it easy to extract the data that you need from a website.
I am currently using both requests
and Beautiful Soup
for my Vault research project, where I am web scraping 3rd party Android app stores to keep an updated list of all available apps.
I am also using these libraries for my IoT research project, where I am web scraping IoT device manufacturing sites to keep an updated list of all manufactured IoT devices.
Both of these projects require me to retrieve and parse data from multiple websites, and the combination of requests
and Beautiful Soup
makes it easy to do so efficiently and effectively.
We'll be learning more about how to use these libraries in Python in this post.
requests
is a library for making HTTP requests in Python.
It allows you to send HTTP requests (such as GET, POST, PUT, DELETE) to a web server and receive a response.
For example:
import requests response = requests.get("http://www.example.com") print(response.status_code) # prints 200 if the request is successful print(response.text) # prints the HTML content of the webpage
Beautiful Soup
is a library for parsing HTML and XML documents. It allows you to extract data from a webpage in a more convenient and efficient way than manually parsing the HTML. For example:
from bs4 import BeautifulSoup html = """ <html> <head> <title>My webpage</title> </head> <body> <h1>Hello, world!</h1> <p>This is my webpage</p> </body> </html> """ soup = BeautifulSoup(html, "html.parser") title = soup.find("title").string print(title) # prints "My webpage"
You can use requests
and Beautiful Soup
together to scrape data from a webpage.
For example:
import requests from bs4 import BeautifulSoup url = "http://www.example.com" response = requests.get(url) soup = BeautifulSoup(response.text, "html.parser") # Extract data from the webpage using Beautiful Soup title = soup.find("title").string paragraphs = soup.find_all("p") # Print the extracted data print(title) for p in paragraphs: print(p.string)
requests
and Beautiful Soup
are just two of the many libraries available for web scraping and parsing in Python.
They are widely used and well-documented, making them a good choice for beginners.
I hope this post has introduced you to the requests
and Beautiful Soup
libraries in Python.
In the next post, we'll look at some more advanced topics in Python.
Thanks for reading!