Jan 12th, 2023
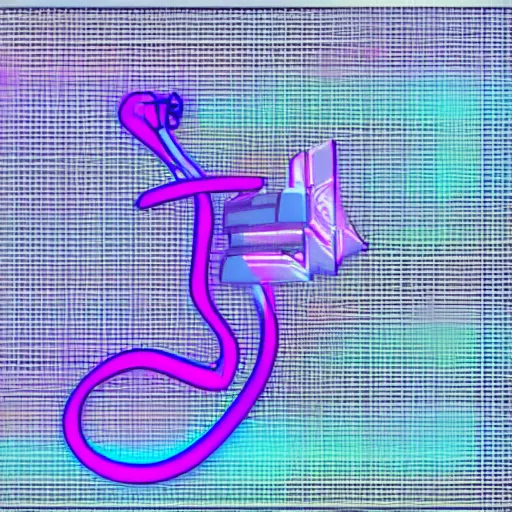
Welcome back to our Python3 intro course! In the last few lessons, we've covered a range of foundational Python concepts, including variables, data types, loops, conditional statements, functions, modules, and file input and output. These are all important concepts to understand as they form the building blocks of any Python program. In this lesson, we'll be exploring some more advanced topics, including exception handling and object-oriented programming.
Exception handling is a technique for handling runtime errors in your code.
When an error occurs, Python raises an exception, which can be caught and handled using the try
and except
statements.
This allows you to gracefully handle errors in your program, rather than having it crash or produce unexpected results.
For example, you might use exception handling to validate user input, to handle network errors when making web requests, or to handle cases where a file is not found.
Exception handling is an important tool to have in your programming toolkit, as it helps you to write more robust and reliable code.
For example:
try: x = 5 / 0 except ZeroDivisionError: print("Cannot divide by zero")
This code will raise a ZeroDivisionError
exception when it attempts to divide 5 by 0, but the exception will be caught and the message "Cannot divide by zero" will be printed instead of the error.
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of "objects", which can contain data and code that operates on that data. In Python, we can create classes to define our own objects. For example:
class Dog: def __init__(self, name, breed): self.name = name self.breed = breed def bark(self): print("Woof!") dog1 = Dog("Fido", "Labrador") print(dog1.name) # prints "Fido" dog1.bark() # prints "Woof!"
In this example, we define a Dog
class with a __init__
method (a special method in Python that is called when an object is created) and a bark
method.
We then create a dog1
object of type Dog
and call its methods.
I hope this gives you a good introduction to exception handling and object-oriented programming in Python. In the next post, we'll dive even deeper into these topics and learn about inheritance and polymorphism. Thanks for reading!