Jan 11th, 2023
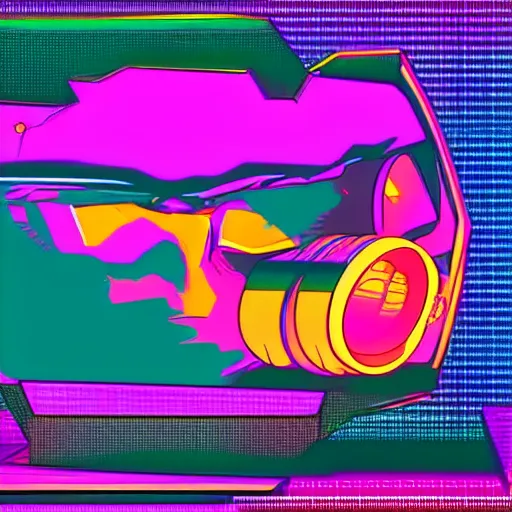
Welcome back to our Python3 intro course! In the last lesson, we learned about using modules and libraries in Python. In this lesson, we'll be exploring how to work with file input and output in Python.
One of the most useful features of programming languages is the ability to read data from and write data to files.
This allows you to store and retrieve data on a permanent basis, rather than just while your program is running.
I find myself using this feature all the time, and the with
function we will cover can also be used for various other functions, like automatically closing a socket either after a transmission is ended or if the connections drops.
This lesson should greatly help with your understanding of these concepts.
In Python, you can read and write files using the built-in open()
function and the read()
, readline()
, write()
, and close()
methods of file objects.
For example:
# Open a file for writing f = open("test.txt", "w") # Write some text to the file f.write("Hello, World!") # Close the file f.close()
This creates a new file called "test.txt" and writes the string "Hello, World!" to it.
You can also open a file for reading using the "r" mode, like this:
# Open a file for reading f = open("test.txt", "r") # Read the contents of the file contents = f.read() # Print the contents print(contents) # Output: Hello, World! # Close the file f.close()
You can use the readline()
method to read a single line of the file at a time, or you can use a for
loop to iterate over the lines of the file.
For example:
# Open a file for reading f = open("test.txt", "r") # Read and print each line of the file for line in f: print(line) # Close the file f.close()
It's important to close your files when you're done with them to free up system resources.
You can use the with
statement to automatically close a file when you're done with it.
For example:
# Open a file for reading with open("test.txt", "r") as f: # Read and print each line of the file for line in f: print(line)
This will automatically close the file when the with
block ends.
I hope this has been a helpful introduction to file input and output in Python. These concepts will be essential as you continue to learn and work with Python, so be sure to practice and get comfortable with them. In the next lesson, we'll be exploring more advanced topics such as exception handling and object-oriented programming. Stay tuned!