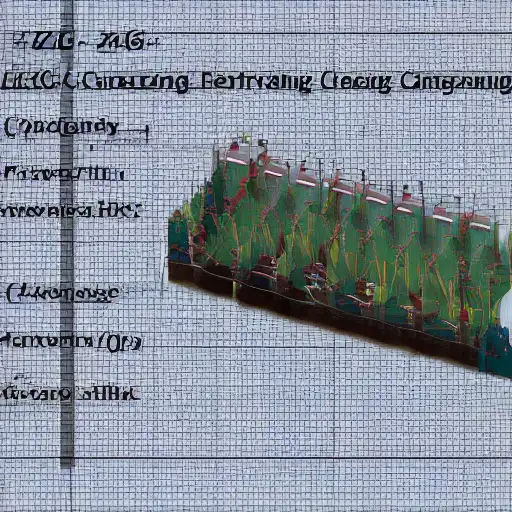
September 24th, 2023
Welcome to the twenty-third installment of our exploration of advanced topics in Zig. In this installment, we have the freedom to choose an advanced topic to delve into, and today, we'll focus on Concurrency in Zig.
Concurrency in Zig
Concurrency is a critical aspect of modern software development, enabling programs to perform multiple tasks simultaneously and efficiently utilize modern hardware with multiple cores and threads. Zig provides robust support for concurrency, making it suitable for building concurrent applications and taking full advantage of multi-core processors. Let's explore some key concepts and techniques for concurrency in Zig:
Coroutines and async
/await
Zig introduces coroutines, a powerful concurrency feature that enables asynchronous programming using the async
and await
keywords. Coroutines allow you to write non-blocking code that can pause and resume execution, making it easier to work with I/O operations and perform concurrent tasks.
Here's a simple example of using async
/await
in Zig:
const std = @import("std"); pub fn main() !void { const result = asyncTask(); try result.await(); std.debug.print("Task completed.\n"); } async fn asyncTask() !void { std.debug.print("Starting async task...\n"); await std.os.sleep(std.duration.seconds(2)); std.debug.print("Async task completed.\n"); }
In this example, the asyncTask
function performs an asynchronous operation, and the main
function awaits its completion, allowing other tasks to run concurrently.
Message-Passing Concurrency
Zig provides a message-passing concurrency model using channels. Channels allow you to send and receive messages between threads or coroutines, facilitating safe communication and synchronization. This model is useful for building concurrent systems where different components need to communicate without shared memory access.
Here's an example of using channels in Zig:
const std = @import("std"); pub fn main() void { const myChannel = std.concurrent.Channel(i32).init(10); // Buffer size 10 const producer = std.spawn(asyncProducer, .{myChannel.writer()}); const consumer = std.spawn(asyncConsumer, .{myChannel.reader()}); std.thread.join(producer); std.thread.join(consumer); } async fn asyncProducer(writer: *std.concurrent.Writer(i32)) !void { for (i in 0..10) { try writer.send(i); } try writer.close(); } async fn asyncConsumer(reader: *std.concurrent.Reader(i32)) !void { while (true) { const value = try reader.receive(); if (value == null) { break; } std.debug.print("Received: {}\n", .{value}); } }
In this example, a producer coroutine sends integers to a channel, and a consumer coroutine receives and processes them concurrently.
Mutexes and Atomics
Zig provides primitives for working with mutexes and atomics to manage shared data and synchronize access to resources. Mutexes allow you to protect critical sections of code, ensuring that only one thread can access them at a time. Atomics provide lock-free operations for variables shared among multiple threads, reducing contention and improving performance.
Here's a simple example of using a mutex in Zig:
const std = @import("std"); pub fn main() void { var sharedValue: i32 = 0; const myMutex = std.concurrent.Mutex.init; const threads: [4]std.Thread = undefined; for (threads) |thread, i| { thread = std.spawn(asyncIncrement, .{&sharedValue, &myMutex}); } for (threads) |thread, i| { std.thread.join(thread); } std.debug.print("Final shared value: {}\n", .{sharedValue}); } async fn asyncIncrement(sharedValue: *volatile i32, mutex: *std.concurrent.Mutex) void { std.withLock(mutex) |lock| { for (0..1000) |i| { sharedValue.* += 1; } } }
In this example, multiple threads increment a shared value while protecting it with a mutex to prevent data races.
Explore More Concurrency
Concurrency is a vast topic with many advanced aspects, including parallelism, thread pools, and advanced synchronization techniques. As you continue your journey in Zig, consider exploring these advanced concurrency topics to build scalable and efficient concurrent applications.
Thank you for joining us on this exploration of advanced topics in Zig. Happy coding!