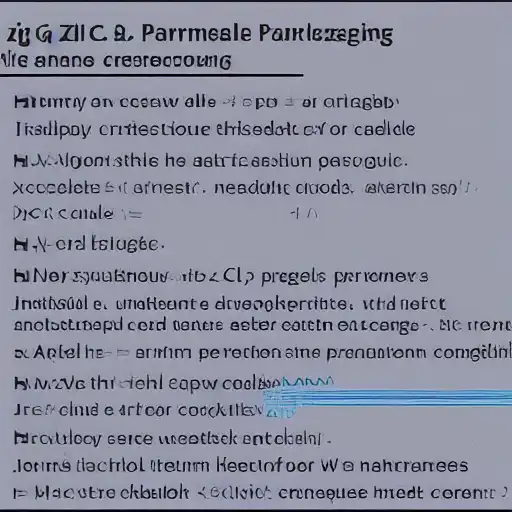
September 25th, 2023
Welcome to the twenty-fourth installment of our exploration of advanced topics in Zig. In this installment, we have the freedom to choose an advanced topic to delve into, and today, we'll focus on Optimizing Performance with Zig.
Optimizing Performance with Zig
Zig is known for its emphasis on performance, and it provides developers with a set of powerful tools and techniques to optimize code for speed and efficiency. Whether you're working on high-performance applications or just want to make your Zig code run as fast as possible, optimizing performance is a crucial skill. Here are some key strategies and techniques for optimizing performance with Zig:
Profiling and Benchmarking
Before you start optimizing your code, it's essential to identify bottlenecks and areas that need improvement. Profiling and benchmarking tools can help you pinpoint performance issues. Zig provides built-in support for benchmarking through the std.testing module. You can write benchmarks to measure the execution time of specific code sections and identify performance hotspots.
Here's a simple example of a Zig benchmark:
const std = @import("std"); test "My Benchmark" { const start = std.time.monotonic(); // Code to benchmark... const end = std.time.monotonic(); const elapsed = end - start; std.testing.expect(elapsed < 1000, "Benchmark took too long"); }
Data Structures and Algorithms
Choosing the right data structures and algorithms is fundamental to achieving optimal performance. Zig provides a rich set of data structures in its standard library, including arrays, slices, maps, and more. By selecting the appropriate data structures and algorithms for your specific use case, you can significantly improve your program's efficiency.
Inline Assembly
For fine-grained control over low-level operations, Zig allows you to use inline assembly. This feature enables you to write assembly code directly within your Zig code, giving you full control over CPU instructions. While it's a powerful tool, inline assembly should be used sparingly and only for performance-critical sections of code.
Here's a simple example of using inline assembly in Zig:
const std = @import("std"); pub fn main() void { var data: u32 = 42; asm volatile { "inc %0" : "+r"(data) }; std.debug.print("Incremented value: {}\n", .{data}); }
Compiler Optimizations
Zig's compiler, zig
, includes various optimization flags that can significantly improve performance. These flags control how the compiler generates machine code and can result in faster execution. Common optimization flags include -O
, -OFast
, and -ORelease
. Be sure to consult the Zig documentation for details on compiler optimization options.
Avoiding Unnecessary Abstractions
While Zig offers high-level abstractions when needed, using them excessively can lead to performance overhead. When optimizing for performance, consider whether certain abstractions can be avoided or replaced with more efficient code. This may involve manual memory management, inlining functions, or eliminating unnecessary checks.
Benchmarking and Profiling Repeatedly
Optimizing code is an iterative process. After making optimizations, it's essential to re-run benchmarks and profiling tools to measure the impact of your changes. This allows you to fine-tune your code and achieve the best possible performance.
Going Beyond
Optimizing performance in Zig is a deep and rewarding journey. Depending on your specific project and requirements, you may explore further topics such as parallelism, SIMD (Single Instruction, Multiple Data) optimizations, and low-level systems programming for ultimate performance gains. The key is to strike a balance between readability and performance while continuously measuring and refining your code.
Thank you for joining us in this exploration of performance optimization in Zig. We hope this installment has provided you with valuable insights into maximizing the efficiency of your Zig programs. Happy coding, and may your Zig applications perform at their best!