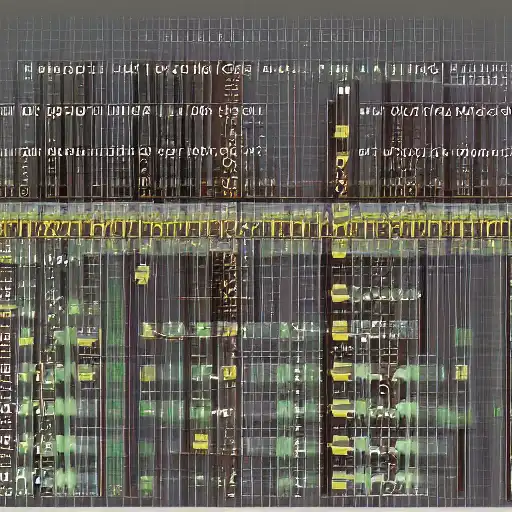
September 23rd, 2023
Welcome to the twenty-second installment of our exploration of advanced topics in Zig. In this installment, we have the freedom to choose an advanced topic to delve into, and today, we'll focus on Low-Level Systems Programming with Zig.
Low-Level Systems Programming with Zig
Zig's design philosophy revolves around providing developers with the tools and capabilities needed for low-level systems programming. Whether you're interested in writing operating systems, device drivers, or just optimizing code for performance, Zig is a powerful language for such tasks. Let's explore some key aspects of low-level systems programming with Zig:
Memory Management
Zig offers fine-grained control over memory management, making it suitable for systems programming where memory efficiency is critical. You can allocate and deallocate memory manually, and Zig provides built-in constructs for managing memory safely.
For example, Zig's allocator
and Allocator
concepts allow you to define custom memory allocators, which is particularly useful when working in resource-constrained environments.
const std = @import("std"); pub fn main() void { const allocator = std.heap.page_allocator; const myBuffer = allocator.alloc(u8, 1024); defer allocator.dealloc(myBuffer); // Work with myBuffer... }
In this example, we allocate a buffer manually from the heap and ensure it's deallocated when it's no longer needed.
Control Over Error Handling
Low-level systems programming often involves handling errors and failures gracefully. Zig's error handling mechanism, including the use of the try
keyword and error sets, allows you to write robust code that can recover from errors and continue execution.
const std = @import("std"); fn potentiallyFailingOperation() !void { if (/* some condition */) { return Error.Generic("Something went wrong"); } // Perform operation... } pub fn main() void { const result = try potentiallyFailingOperation(); if (result instanceof Error) { std.debug.print("Error: {}\n", .{result}); // Handle the error... } else { // Continue execution... } }
In this example, we define a function that may return an error, and we handle it appropriately in the calling code.
Low-Level Programming Constructs
Zig provides low-level programming constructs like inline assembly, volatile loads and stores, and direct control over CPU registers. These features allow you to write code that interfaces directly with hardware or performs highly optimized operations.
const std = @import("std"); pub fn main() void { var data: u32 = 42; asm volatile { "inc %0" : "+r"(data) }; std.debug.print("Incremented value: {}\n", .{data}); }
In this example, we use inline assembly to increment the data
variable directly.
Interoperability with C
Zig's interoperability with C is seamless, allowing you to leverage existing C libraries and code in your Zig projects. You can call C functions, use C data types, and interface with C libraries directly from Zig.
Here's an example of calling a C function from Zig:
const std = @import("std"); const c = @cImport({ @cInclude("stdio.h"); pub const printf: fn(format: [*]const u8, ...) c.int; }); pub fn main() void { const message = "Hello, Zig!"; c.printf("Message: %s\n", message); }
In this example, we import the printf
function from the C standard library and use it to print a message.
Explore More Low-Level Systems Programming
Low-level systems programming with Zig opens the door to a wide range of possibilities, from creating custom operating systems to optimizing performance-critical code. If you're interested in this advanced topic, consider exploring further in areas such as:
- Operating Systems Development: Zig's low-level capabilities make it an excellent choice for building custom operating systems or kernel modules.
- Device Drivers: Writing device drivers often requires interfacing directly with hardware, and Zig's low-level features are well-suited for this task.
- Embedded Systems: Zig's minimal runtime and control over memory allocation make it a great choice for embedded systems programming.
- Performance Optimization: Zig allows you to write highly optimized code, making it suitable for tasks where performance is paramount.
- Security Research: Zig's focus on safety and predictability makes it an attractive language for security researchers and exploit developers.
Thank you for joining us on this exploration of advanced topics in Zig. Happy coding!