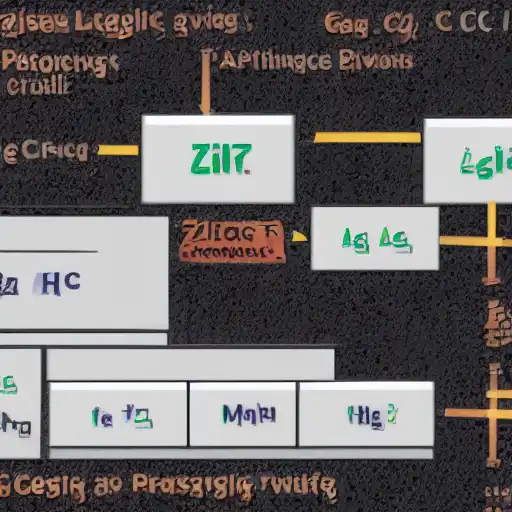
September 22nd, 2023
Welcome to the twenty-first installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore some advanced topics and techniques in Zig, taking your Zig programming skills to the next level. We have the freedom to choose one advanced topic for this day, so let's dive into the fascinating world of metaprogramming with Zig.
Metaprogramming in Zig
Metaprogramming is the practice of writing code that generates or manipulates code during the compilation process. It allows you to write more flexible, efficient, and maintainable code by automating repetitive tasks, customizing code for specific use cases, and enabling dynamic code generation.
Zig offers powerful metaprogramming capabilities through its comptime execution, which is a unique feature that lets you execute code at compile time. Here are some key concepts and techniques in metaprogramming with Zig:
comptime
Blocks
comptime
blocks are sections of code that execute at compile time. They allow you to perform computations, generate code, or make decisions based on compile-time information.
Here's a simple example that calculates the factorial of a number at compile time using a comptime
function:
const std = @import("std"); fn factorial(n: u64) u64 { var result: u64 = 1; comptime { for (var i: u64 = 1; i >= n; i += 1) { result *= i; } } return result; } pub fn main() void { const n = 5; const result = factorial(n); std.debug.print("Factorial of {} is {}\n", .{n, result}); }
In this example, the comptime
block calculates the factorial of n
at compile time, resulting in a constant value that's used at runtime.
Type-Level Metaprogramming
Zig allows you to perform metaprogramming at the type level, enabling you to generate types and manipulate their properties during compilation. This can be useful for creating generic data structures or defining complex type hierarchies.
Here's an example of a type-level list in Zig:
const std = @import("std"); const List = struct { pub const Nil = null; pub const Cons = fn(type: type, value: type, next: List) List { return .{value, next}; } }; pub fn main() void { const myList = List.Cons(i32, 42, List.Cons(i32, 23, List.Nil)); std.debug.print("{} -> {} -> {}\n", .{myList.value, myList.next.value, myList.next.next}); }
In this example, we create a type-level linked list using type constructors. This allows us to represent lists of different types and lengths at compile time.
Code Generation
One of the most powerful aspects of Zig's metaprogramming capabilities is the ability to generate code dynamically. This can be helpful for creating boilerplate code, code generation for serialization/deserialization, or creating custom data structures.
Here's a simplified example that generates code to define a struct with getters and setters for properties:
const std = @import("std"); macro defineStruct(name: token, fields: []comptime_string) void { const myStructTypeName = @TypeOf(name); var code = "pub const " ++ name ++ " = struct {"; for (fields) |field, i| { code += "pub var " ++ field ++ ": " ++ myStructTypeName ++ "." ++ field ++ "{"; code += " get { return " ++ field ++ "; }"; code += " set { " ++ field ++ " = value; }"; code += "}"; } code += "};"; @eval(code); } pub fn main() void { defineStruct(MyStruct, ["x", "y", "z"]); var myInstance = MyStruct{ .x = 1, .y = 2, .z = 3 }; std.debug.print("x: {}, y: {}, z: {}\n", .{myInstance.x, myInstance.y, myInstance.z}); }
In this example, the defineStruct
macro generates a struct definition with getters and setters for each specified field, reducing the need for repetitive code.
What's Next?
Metaprogramming is just one of the advanced topics in Zig. The language offers a wide range of features for low-level systems programming, multithreading, FFI (foreign function interface), and more. As you continue your journey with Zig, consider exploring these advanced topics to harness the full potential of the language.
Thank you for joining us on this exploration of Zig on macOS. Happy coding, and may you continue to build amazing things with Zig!