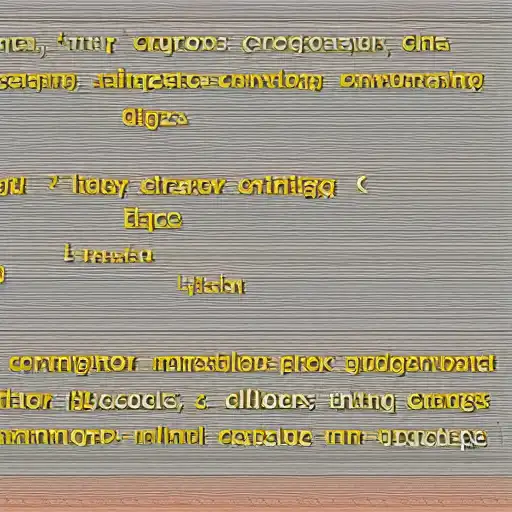
September 21st, 2023
Welcome to the twentieth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore cross-platform development with Zig, allowing you to develop applications that run on multiple operating systems effortlessly.
The Power of Cross-Platform Development
Cross-platform development refers to the practice of creating software applications that can run on multiple operating systems with minimal modifications. It's a valuable approach because it allows developers to reach a broader audience and maintain a single codebase.
Zig, with its focus on portability, low-level programming, and strong emphasis on cross-compilation, is well-suited for cross-platform development. Here's how you can leverage Zig for this purpose:
Compiling and Targeting Different OSes
Zig's cross-compilation capabilities enable you to compile your code for various target operating systems. You can specify the target platform and architecture using the zig build
command, and Zig will generate binaries tailored to those specifications.
For example, to compile a Zig program for Linux and Windows, you can use the following commands:
zig build -Dtarget=x86_64-linux zig build -Dtarget=x86_64-windows
Zig can target a wide range of operating systems, including macOS, Linux, Windows, Android, iOS, and more. By specifying the target, you ensure that the generated binary is compatible with the chosen platform.
Platform-Dependent Code
In cross-platform development, you may encounter situations where specific code should run only on certain platforms. Zig provides platform-specific compilation blocks to handle these cases.
Here's an example of platform-dependent code in Zig:
const os = @import("std").os; pub fn main() void { // Common code for all platforms if (os(target_os) == .windows) { // Code specific to Windows } else if (os(target_os) == .linux) { // Code specific to Linux } else if (os(target_os) == .macos) { // Code specific to macOS } // More common code }
In this example, we use the os(target_os)
function to conditionally execute code based on the target operating system.
Libraries and Dependencies
When developing cross-platform applications in Zig, consider the libraries and dependencies you use. Ensure that the libraries you rely on are also cross-platform compatible or have platform-specific implementations when necessary.
Additionally, Zig's package manager, zigmod
, allows you to specify dependencies and versions in your project's zig.mod
file, making it easier to manage external libraries and ensure compatibility across platforms.
Testing on Different Platforms
To ensure that your cross-platform application works correctly on various operating systems, it's essential to test it thoroughly. Set up testing environments for each target platform, and use automated testing tools to detect platform-specific issues.
Continuous integration (CI) services can help automate the testing process, allowing you to run tests on multiple platforms with ease.
User Experience and Design
Consider the user experience and design aspects when developing cross-platform applications. Each operating system has its own design guidelines and user interface conventions. Adapting your application's user interface to the platform can enhance the user experience and make it feel more native.
What's Next?
In this final part, you've learned about cross-platform development with Zig, allowing you to create applications that can run seamlessly on multiple operating systems. As you embark on your journey in cross-platform development with Zig, remember to test rigorously, adapt your user interface, and consider the nuances of each target platform to create a cohesive and user-friendly experience. Happy coding, and may your cross-platform applications reach a wide and diverse audience!