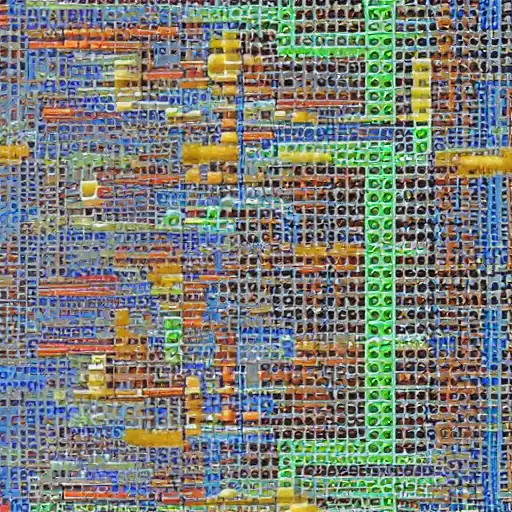
September 20th, 2023
Welcome to the nineteenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore essential debugging and profiling techniques in Zig, helping you identify and resolve issues in your code while optimizing performance.
Debugging Techniques in Zig
Debugging is a critical part of software development, and Zig provides several tools and techniques to help you find and fix issues in your code.
Printing Debug Messages
The simplest debugging technique is using print statements to output values, variables, or messages to the console. Zig's standard library includes the std.debug
module, which provides functions like print
, println
, and print
for displaying debug information.
Here's an example:
const std = @import("std"); fn main() void { const message = "Debugging in Zig!"; std.debug.print("Message: {}\n", .{message}); const value = 42; std.debug.print("Value: {}\n", .{value}); }
By using print statements strategically, you can inspect variables and program flow to understand what's happening in your code.
Assertions
Assertions are checks placed in the code to verify that certain conditions hold true. If an assertion fails, it typically indicates a bug in the code. Zig provides the @assert
function for this purpose.
Here's an example:
const std = @import("std"); fn main() !void { const value = 42; @assert(value > 0, "Value must be positive"); // Rest of the code... }
If value
is not greater than 0, the program will terminate with an assertion error message.
Debugger
Zig has a built-in debugger called zig build --debug
. You can compile your code with the --debug
flag, and then use the lldb
debugger to step through the code, set breakpoints, and inspect variables.
For example, to debug a Zig program called my_program.zig
, you can use:
zig build --debug lldb ./zig-cache/my_program
The debugger allows you to interactively examine and modify the program's state.
Profiling for Performance Optimization
Profiling helps you identify bottlenecks and performance issues in your code. Zig provides tools for profiling your programs.
Benchmarking
You can use the std.testing
module to write benchmarks for your code. Benchmarks measure the execution time of specific code sections. By identifying the slowest parts of your program, you can focus your optimization efforts where they will have the most impact.
Here's a simple benchmark example:
const std = @import("std"); test "My Benchmark" { const start = std.time.monotonic(); // Code to benchmark... const end = std.time.monotonic(); const elapsed = end - start; std.testing.expect(elapsed < 1000, "Benchmark took too long"); }
Profiling Tools
Zig can interface with profiling tools like perf
(Linux), Instruments
(macOS), and VTune
(Intel's VTune Profiler) for detailed performance analysis. These tools provide insights into CPU usage, memory usage, and more.
To use profiling tools with Zig, you typically compile your program with appropriate flags and then analyze the generated profiles.
Tips for Troubleshooting
Here are some additional tips for troubleshooting and debugging in Zig:
- Use Version Control: Keep your codebase under version control (e.g., Git) to track changes and easily revert to a known good state if needed.
- Unit Tests: Write unit tests to ensure that individual components of your code work as expected. This can help catch issues early.
- Review Documentation: Consult Zig's documentation and the documentation for any libraries or tools you're using to understand their behavior and potential issues.
- Pair Programming: Collaborating with a colleague on debugging can provide fresh insights and different perspectives.
- Keep It Simple: Simplify your code to isolate issues. Remove unnecessary complexity to make it easier to identify problems.
- Rubber Duck Debugging: Explaining your code and problem to someone else (or even an inanimate object like a rubber duck) can help you think through the issue logically.
- Learn from Errors: When you encounter errors or issues, take the time to understand what caused them. This knowledge can prevent similar problems in the future.
What's Next?
In this part, you've learned about debugging and profiling techniques in Zig, crucial skills for identifying and resolving issues in your code while optimizing performance. As you continue your journey in Zig development, practice these techniques to become a more effective troubleshooter and developer. Happy coding!