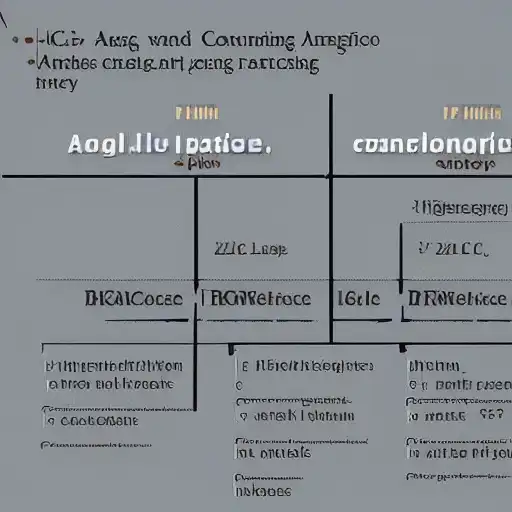
September 19th, 2023
Welcome to the eighteenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore Zig's role in web development, covering both server-side and client-side web applications, as well as web frameworks and tooling.
Zig's Role in Web Development
Zig is primarily designed as a systems programming language, making it a strong choice for low-level tasks like operating system development, game engines, and system utilities. However, it can also play a role in web development, albeit with some caveats.
Server-Side Web Applications
Zig can be used to build server-side web applications by leveraging its capabilities in networking and concurrency. You can create web servers that handle HTTP requests, process data, and generate dynamic content. While Zig doesn't have a dedicated web framework like other languages (e.g., Django for Python or Express for Node.js), you can build your own using Zig's libraries or interface with C-based web frameworks.
Here's a basic example of a Zig HTTP server:
const std = @import("std"); const http = @import("std").http; pub fn main() !void { const listener = try http.listen(":8080"); defer listener.close(); while (true) { const conn = try listener.accept(); const writer = conn.writer(); const response = http.Response.init(writer); response.status = .Ok; response.setBody("Hello, Zig!"); try response.send(); } }
In this example, we create a simple HTTP server that listens on port 8080 and responds with "Hello, Zig!" to incoming requests.
Client-Side Web Applications
For client-side web applications, Zig's role is more limited. Web development for the browser typically involves languages like HTML, CSS, and JavaScript. While it's possible to compile Zig to WebAssembly (Wasm) and use it in the browser, this approach is less common compared to using JavaScript or other languages specifically designed for browser scripting.
Zig's strength in web development often shines when you're dealing with WebAssembly-based applications or integrating with existing web services through APIs. You can write Zig code to interact with the browser's Web API or work with JavaScript libraries through WebAssembly.
Web Frameworks and Tooling
Zig does not have as mature and well-established web development frameworks and tooling as other languages like JavaScript, Python, or Ruby. However, Zig's active community is continuously working on developing new libraries and tools.
When working on web projects with Zig, you may need to build some components from scratch or interface with C libraries and tools. Keep an eye on Zig's official repositories, community-contributed projects, and forums for updates and new developments in the web development space.
What's Next?
In this part, you've explored Zig's role in web development, including its use in server-side and client-side web applications. While Zig may not have as extensive a web development ecosystem as other languages, its capabilities in networking and low-level programming make it a unique choice for certain web-related tasks.
As you dive deeper into web development with Zig, consider exploring Zig's growing ecosystem of web-related libraries and tools, and keep an eye on the language's development to see how it evolves in the web development space. Happy coding!