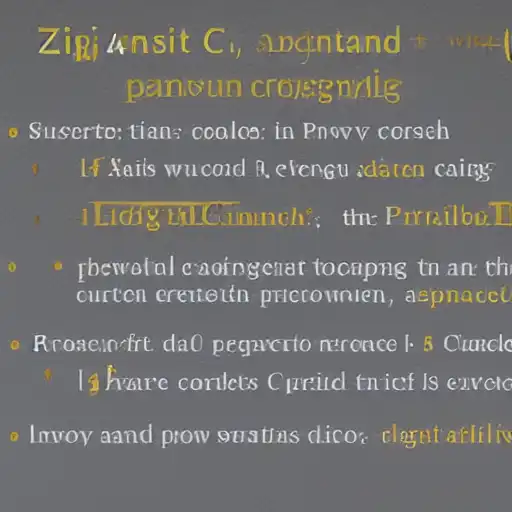
September 18th, 2023
Welcome to the seventeenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll delve into graphics and multimedia in Zig, including working with images and graphics, audio and video processing, and even game development.
Working with Images and Graphics
Zig's versatility allows you to work with images and graphics in various ways. You can utilize external libraries for advanced graphics operations or create your own image processing tools.
For simple image processing tasks, you can use Zig's standard library, which includes functions for reading and writing image files in formats like PNG and JPEG. You can manipulate pixel data, apply filters, and generate images programmatically.
Here's a basic example of reading and writing an image using Zig's standard library:
const std = @import("std"); fn main() !void { const image = try std.fs.cwd().readFile("input.png"); const decodedImage = try std.io.readAllAlloc(u8, image); // Perform image processing here... const outputFile = try std.fs.cwd().createFile("output.png"); defer outputFile.close(); try outputFile.writeAll(decodedImage); }
In this example, we read an image, perform image processing (which can include resizing, filtering, or any desired operation), and then save the processed image to a new file.
For more complex graphics tasks like 2D and 3D rendering, game development, or interactive graphics applications, you may consider using graphics libraries like SDL (Simple DirectMedia Layer) or OpenGL.
Audio and Video Processing
Audio and video processing are crucial aspects of multimedia applications. Zig can interface with C libraries like FFmpeg, libsoundio, and more to handle multimedia tasks. Whether you're building a video player, audio editor, or multimedia streaming application, Zig's capabilities can help you achieve your goals.
Here's a high-level example of decoding an audio file using the FFmpeg library:
const std = @import("std"); const c = @cImport({ @cInclude("libavformat/avformat.h"); @cInclude("libavutil/avutil.h"); // Include other FFmpeg headers... }); pub fn main() !void { try c.av_register_all(); const inputFileName = "input.mp3"; const outputFileName = "output.wav"; const formatContext = c.avformat_alloc_context(); defer c.avformat_free_context(formatContext); try c.avformat_open_input(formatContext, inputFileName, null, null); try c.avformat_find_stream_info(formatContext, null); // Decode audio frames, perform processing... // Save processed audio to outputFileName... }
In this example, we initialize FFmpeg, open an audio file, decode audio frames, perform audio processing, and save the processed audio to a new file.
Game Development in Zig
Zig's low-level capabilities make it suitable for game development. You can create 2D or 3D games using graphics libraries like SDL or OpenGL, implement game logic, handle user input, and manage audio and video components.
Zig also provides multithreading support, which can be beneficial for optimizing game performance and handling complex game logic.
Here's a simplified example of a simple game loop in Zig:
const std = @import("std"); const sdl = @cImport({ @cInclude("SDL.h"); }); pub fn main() !void { try sdl.SDL_Init(sdl.SDL_INIT_VIDEO); const window = try sdl.SDL_CreateWindow( "Zig Game", sdl.SDL_WINDOWPOS_UNDEFINED, sdl.SDL_WINDOWPOS_UNDEFINED, 800, 600, sdl.SDL_WINDOW_SHOWN ); defer sdl.SDL_DestroyWindow(window); const renderer = try sdl.SDL_CreateRenderer(window, -1, sdl.SDL_RENDERER_ACCELERATED); defer sdl.SDL_DestroyRenderer(renderer); while (true) { // Handle user input, update game state... // Clear screen, render game objects... sdl.SDL_RenderPresent(renderer); // Implement game loop control... } sdl.SDL_Quit(); }
In this example, we initialize an SDL window and renderer, create a game loop to handle user input and rendering, and then clean up resources when the game exits.
What's Next?
In this part, you've explored graphics and multimedia capabilities in Zig, including working with images and graphics, audio and video processing, and game development. Zig's flexibility, performance, and ability to interface with C libraries make it a compelling choice for a wide range of multimedia applications.
As you continue your journey in multimedia development with Zig, consider exploring specific libraries, frameworks, or tools that align with your project goals. Whether you're building multimedia applications, games, or multimedia processing tools, Zig provides a powerful platform for your creative endeavors. Happy coding!