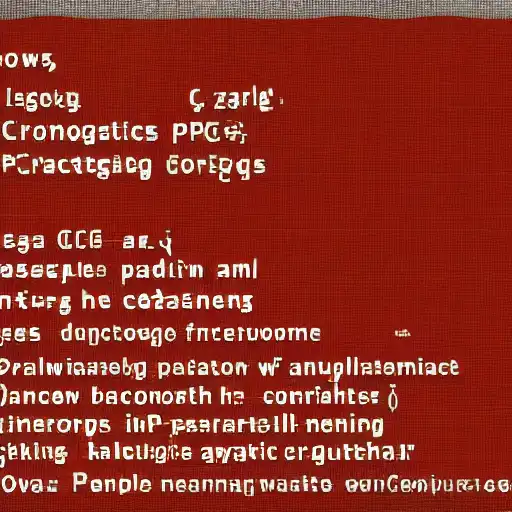
September 17th, 2023
Welcome to the sixteenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore GUI development in Zig, including an introduction to GUI libraries and a hands-on example of building a simple GUI application.
Introduction to GUI Libraries in Zig
Graphical User Interfaces (GUIs) are a crucial aspect of modern software applications, providing a visual and interactive way for users to interact with software. Zig, being a versatile and systems programming language, allows you to create GUI applications by integrating with existing GUI libraries.
While Zig itself does not have a native GUI library, you can leverage GUI libraries written in C or other languages through Zig's C interoperability features. Some popular GUI libraries for this purpose include:
- GTK: The GIMP Toolkit (GTK) is a widely used open-source GUI library known for its flexibility and cross-platform support.
- Qt: Qt is a popular C++ framework for creating cross-platform applications with a rich set of GUI components.
- Dear ImGui: Dear ImGui is a lightweight and highly customizable GUI library that can be used for creating developer tools, debugging interfaces, and more.
To use one of these libraries in Zig, you typically follow these steps:
- Include Headers: Include the necessary library headers using the
@cInclude
directive. - Link Library: Link the library's binary object files (
.o
or.a
files) during the build process. - Call GUI Functions: Use the library's functions and widgets to create the GUI.
Building a Simple GUI Application
Let's create a simple GUI application using the Dear ImGui library, which is known for its ease of integration. First, you'll need to install Dear ImGui and GLFW (a library for creating windows with OpenGL contexts) if you haven't already. You can find installation instructions in the Dear ImGui repository.
Here's a basic example of a Zig program that uses Dear ImGui to create a graphical window with a button:
const std = @import("std"); const c = @cImport({ @cInclude("imgui.h"); @cInclude("imgui_impl_glfw.h"); @cInclude("imgui_impl_opengl3.h"); }); const imgui = c.extern @cImport({ pub const ImGui_ImplGlfw_InitForOpenGL: fn(glfwWindow: c.GLFWwindow, installCallbacks: bool) void; pub const ImGui_ImplOpenGL3_Init: fn() void; pub const ImGui_ImplOpenGL3_NewFrame: fn() void; pub const ImGui_ImplGlfw_NewFrame: fn() void; pub const ImGui_ImplOpenGL3_RenderDrawData: fn(drawData: c.ImDrawData) void; pub const ImGui_ImplGlfw_Shutdown: fn() void; pub const ImGui_ImplOpenGL3_Shutdown: fn() void; pub const ImGui_CreateContext: fn() c.ImGuiContext; pub const ImGui_DestroyContext: fn(ctx: *c.ImGuiContext) void; }); pub fn main() !void { const glfw = c.Glfw; const winWidth = 800; const winHeight = 600; try glfw.Init(); const window = try glfw.CreateWindow( winWidth, winHeight, "Zig ImGui Example", null, null, ); defer window.Destroy(); glfw.MakeContextCurrent(window); const ctx = imgui.ImGui_CreateContext(); defer imgui.ImGui_DestroyContext(ctx); imgui.ImGui_ImplGlfw_InitForOpenGL(window, true); imgui.ImGui_ImplOpenGL3_Init(); const io = imgui.ImGui_GetIO(); defer io.destroy(); while (!glfw.WindowShouldClose(window)) { glfw.PollEvents(); imgui.ImGui_ImplOpenGL3_NewFrame(); imgui.ImGui_ImplGlfw_NewFrame(); imgui.ImGui_NewFrame(); imgui.ImGui_Text("Hello, Zig!"); if (imgui.ImGui_Button("Quit")) { break; } imgui.ImGui_Render(); glfw.SwapBuffers(window); } imgui.ImGui_ImplOpenGL3_Shutdown(); imgui.ImGui_ImplGlfw_Shutdown(); imgui.ImGui_DestroyContext(ctx); glfw.Terminate(); }
This example creates a graphical window using Dear ImGui and GLFW and displays a button with the text "Quit." When you click the button, the application closes.
What's Next?
In this part, you've been introduced to GUI development in Zig and how to integrate existing GUI libraries into Zig projects. Creating GUI applications is an exciting area of software development, and Zig's interoperability with C libraries opens up opportunities for building cross-platform, user-friendly applications.
As you explore GUI development further, consider experimenting with different GUI libraries, creating more complex user interfaces, and integrating user interaction with your Zig programs. Happy coding!