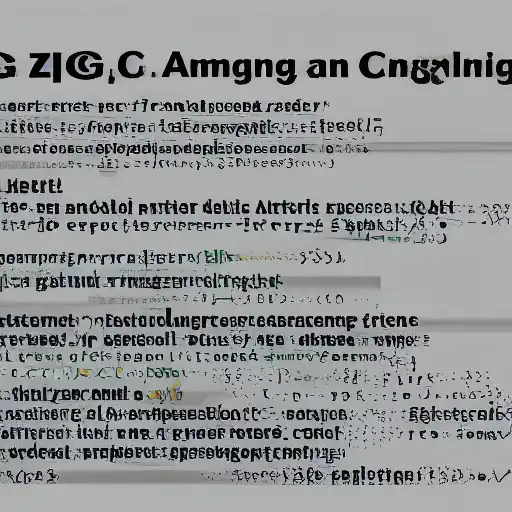
September 16th, 2023
Welcome to the fifteenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore networking in Zig, covering socket programming, building HTTP clients and servers, working with WebSockets, and discussing networking best practices.
Socket Programming with Zig
Zig provides powerful capabilities for socket programming, allowing you to create networked applications with ease. You can use the std.net
module to work with sockets and network protocols. Here's a basic example of creating a TCP server in Zig:
const std = @import("std"); fn main() !void { const address = try std.net.Address.parseIp4("127.0.0.1", 8080); const listener = try std.net.tcpListen(address, .{}); defer listener.close(); while (true) { const conn = try listener.accept(); const connWriter = conn.writer(); try connWriter.print("Hello, Zig!\n"); } }
In this example, we create a TCP server that listens on the local address (127.0.0.1
) and port 8080
. When a client connects, we send a "Hello, Zig!" message to the client.
HTTP Client and Server Examples
Building HTTP clients and servers is a common use case in networking applications. Zig's std.net.http
module simplifies working with HTTP requests and responses. Here's an example of an HTTP server in Zig:
const std = @import("std"); fn main() void { const server = std.net.http.Server.init(); server.handle(std.net.http.Method.GET, "/hello", |_, resp| { resp.write("Hello, Zig!\n"); }); server.serve(":8080"); }
In this example, we create an HTTP server that responds to GET requests to the "/hello" endpoint with a "Hello, Zig!" message.
To create an HTTP client, you can use the std.net.http.Client
type to make requests and handle responses. Here's a simple HTTP client example:
const std = @import("std"); fn main() void { const client = std.net.http.Client.init(); const request = client.get("http://example.com"); const response = try request.perform(); std.debug.print("Response: {}\n", .{response.body}); }
In this example, we make an HTTP GET request to "http://example.com" and print the response body.
WebSockets and Networking Best Practices
Zig also supports WebSockets through the std.net.ws
module, making it easy to build real-time applications that require bidirectional communication. WebSockets provide a low-latency, efficient way to exchange data between clients and servers.
When working with networking in Zig, it's essential to follow best practices, including:
- Error handling: Always handle errors gracefully and provide meaningful error messages to users.
- Security: Be aware of security best practices, such as validating input and using secure communication protocols (e.g., TLS).
- Efficiency: Optimize network communication for performance by minimizing data transfer and reducing latency.
- Scalability: Design your networked applications to scale efficiently as the user base grows.
What's Next?
In this part, you've learned about networking in Zig, including socket programming, building HTTP clients and servers, and working with WebSockets. Networking is a critical skill for building modern applications, and Zig provides the tools you need to create robust networked applications.
As you continue to explore Zig, consider building networked applications and services to gain hands-on experience in this important domain. Networking knowledge is valuable for a wide range of software development roles and projects. Happy coding!