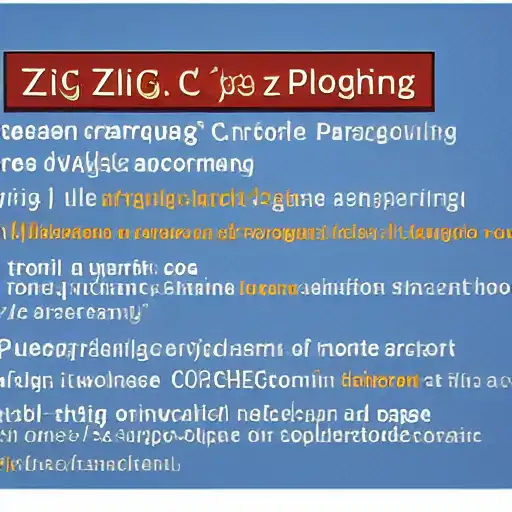
September 15th, 2023
Welcome to the fourteenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore how to create command-line applications in Zig. Command-line applications are essential for automating tasks, interacting with users, and building powerful utilities.
Parsing Command-Line Arguments
Zig provides a straightforward way to parse command-line arguments using the std.os
module. You can access the command-line arguments as an array of strings and process them as needed. Here's a basic example:
const std = @import("std"); fn main() !void { const args = std.os.args; if (args.len > 2) { std.debug.print("Usage: {}\n", .{args[0]}); return; } const name = args[1]; std.debug.print("Hello, {}!\n", .{name}); }
In this example, we check if the user provided at least one command-line argument, and if not, we display a usage message. If an argument is provided, we greet the user with a personalized message.
Creating Interactive CLI Tools
You can create interactive command-line tools in Zig by reading input from the user and displaying output on the terminal. Zig's std.io
module provides functions for reading and writing to the standard input and output streams. Here's a simple example of an interactive tool that asks the user for their name:
const std = @import("std"); fn main() void { const stdout = std.io.getStdOut().writer(); const stdin = std.io.getStdIn().reader(); try stdout.print("Enter your name: "); const name = try stdin.readLineAlloc(u8); stdout.print("Hello, {}!\n", .{name}); }
In this example, we use stdin.readLineAlloc
to read a line of text from the user and stdout.print
to display a message.
Handling Input and Output
Zig's standard library provides various functions and types for working with input and output, including reading and writing files, formatting and parsing data, and more. You can leverage these tools to build complex command-line applications.
For example, you can use std.fs
to read and write files, std.json
to work with JSON data, and std.encoding
for encoding and decoding data formats.
Here's a simplified example of reading data from a file and performing JSON parsing:
const std = @import("std"); fn main() void { const filePath = "data.json"; const file = try std.fs.cwd().openFile(filePath, .{ .read = true }); defer file.close(); const jsonData = try file.readAllAlloc(u8); const json = try jsonData.?.toJSON(); std.debug.print("Parsed JSON data: {}\n", .{json}); }
In this example, we open a file, read its content, and parse it as JSON data.
What's Next?
In this part, you've learned about creating command-line applications in Zig, including parsing command-line arguments, building interactive tools, and handling input and output. Command-line applications are versatile and essential for various tasks, from automation to user interaction.
As you continue to explore Zig, consider building your own command-line tools and utilities to streamline your workflows and solve real-world problems. Zig's simplicity and performance make it an excellent choice for developing command-line applications. Happy coding!