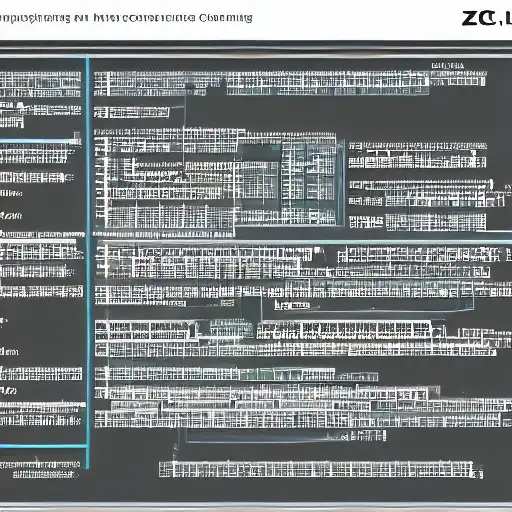
September 14th, 2023
Welcome to the thirteenth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore the topic of interoperability in Zig, which involves using C libraries in Zig, leveraging Zig's C-compatible ABI, and calling Zig code from other programming languages.
Using C Libraries in Zig
Zig has strong support for interoperability with C libraries, making it easy to integrate existing C code into your Zig projects. To use a C library in Zig, you typically follow these steps:
- Include Headers: Include the necessary C library headers using the
@cInclude
directive. - Link C Libraries: Link the C library's binary object files (
.o
or.a
files) during the build process. - Declare C Functions: Declare C functions in Zig using the
@cImport
directive to access them from Zig code.
Here's a simplified example of using the C standard library's printfprintf
function in Zig:
const std = @import("std"); const libc = @cImport({ @cInclude("stdio.h"); }); fn main() void { const message = "Hello, Zig!\n"; libc.printf("%s", .{@cstring(message)}); }
In this example, we import the C standard library's printf
function and use it to print a message.
Zig's C-Compatible ABI
Zig is designed to have a C-compatible ABI, which means you can call Zig functions from C and vice versa. This compatibility simplifies integration with other languages and systems that use C as a common interface.
To expose Zig functions for use in C code, you can mark them with the @cDefine
attribute. For example:
const std = @import("std"); @cDefine pub fn add(a: i32, b: i32) i32 { return a + b; }
This allows you to call the add
function from C code as if it were a regular C function.
Calling Zig from Other Languages
Zig's C-compatible ABI also enables you to call Zig code from other programming languages like C, C++, and even languages with foreign function interfaces (FFI). To do this, you need to create a Zig library that exports functions using the @cDefine
attribute and then link it with the target language.
Here's an example of using a Zig function from a C program:
const std = @import("std"); @cDefine pub fn zig_add(a: i32, b: i32) i32 { return a + b; } int main() { int result = zig_add(3, 4); printf("Result: %d\n", result); return 0; }
In this example, we define a Zig function zig_add
and call it from a C program.
What's Next?
In this part, you've learned about interoperability in Zig, including using C libraries in Zig, Zig's C-compatible ABI, and calling Zig code from other programming languages. Zig's compatibility with C opens up a wide range of possibilities for integrating existing codebases, libraries, and languages into your Zig projects.
As you continue to explore Zig, consider how these interoperability features can help you leverage the extensive ecosystem of C libraries and work seamlessly with other programming languages. Happy coding!