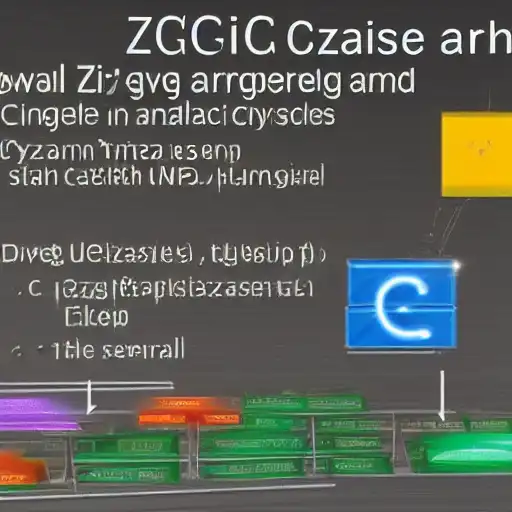
September 13th, 2023
Welcome to the twelfth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore file I/O in Zig, a fundamental aspect of working with data and managing file systems. Zig provides powerful and straightforward tools for reading and writing files, working with directories, and performing serialization and deserialization operations.
Reading and Writing Files
Zig simplifies reading and writing files with its standard library. You can use the std.fs
module to interact with files. Here's an example of reading data from a file:
const std = @import("std"); fn main() !void { const filePath = "example.txt"; const file = try std.fs.cwd().openFile(filePath, .{ .read = true }); defer file.close(); const buffer = try file.readAllAlloc(u8); std.debug.print("Read {} bytes from file:\n{}\n", .{buffer.len, buffer}); }
In this example:
- We use
std.fs.cwd().openFile
to open a file for reading. - After reading the file, we close it using
defer
to ensure proper cleanup. - The content is read into a buffer, which we then print to the console.
openFile
, specifying the write
flag.
Working with Directories
Zig provides functionality for working with directories through the std.fs
module as well. You can create, delete, and manipulate directories easily. Here's an example of creating a directory:
const std = @import("std"); fn main() void { const dirPath = "my_directory"; const result = std.fs.cwd().createDirectory(dirPath, .{}); switch (result) { true => std.debug.print("Directory '{}' created successfully.\n", .{dirPath}), false => std.debug.print("Failed to create directory '{}'.\n", .{dirPath}), } }
In this example, we use createDirectory
to create a directory named "my_directory." You can perform similar operations like removing directories and listing their contents.
Serialization and Deserialization
Zig provides facilities for serialization and deserialization, allowing you to work with structured data formats like JSON and binary data. The standard library includes the std.json
module for JSON operations and the std.mem
module for binary data manipulations.
Here's a simple example of serializing and deserializing JSON data:
const std = @import("std"); fn main() void { const std.json = @import("std").json; const myData = struct { name: []const u8 = "Alice", age: u32 = 30, }; const json = try myData.?.toJson(); std.debug.print("JSON: {}\n", .{json}); const deserialized = try json.?.fromJson(typeof(myData)); std.debug.print("Deserialized: name={}, age={}\n", .{ deserialized.name, deserialized.age, }); }
In this example, we define a struct, serialize it to JSON, and then deserialize it back to the original struct.
What's Next?
In this part, you've learned about file I/O in Zig, including reading and writing files, working with directories, and performing serialization and deserialization operations. These are essential skills for working with data and managing file systems in your Zig applications.
As you continue your Zig journey, you can explore more advanced topics, such as network programming, interfacing with external libraries, and building full-fledged applications. Zig's versatility and performance make it an excellent choice for a wide range of programming tasks. Happy coding!