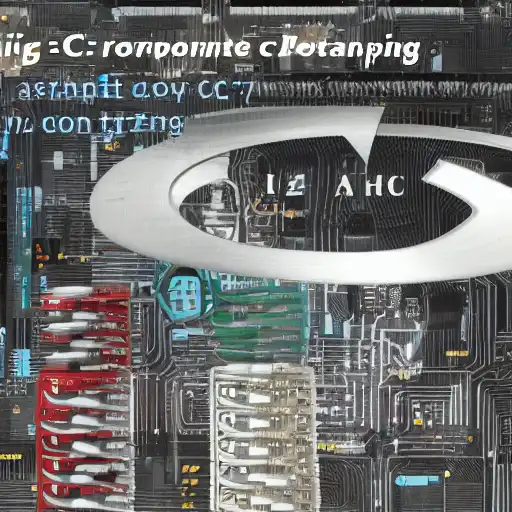
September 4th, 2023
Welcome to the third installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore the fundamental concepts of variables and data types in the Zig programming language. Understanding how to declare variables and work with various data types is crucial as it forms the foundation of any programming language.
Declaring Variables
In Zig, you declare variables using the var
keyword followed by the variable name and its type. Here's a basic example:
var age: i32 = 25;
In this example:
var
is used to declare a variable.age
is the variable name.i32
is the data type, representing a 32-bit signed integer.= 25
initializes the variable with the value 25.
You can also omit the data type and let Zig infer it based on the assigned value:
var name = "John";
In this case, Zig automatically assigns the data type []const u8
(a null-terminated byte array) to the variable name
because it's initialized with a string.
Numeric and String Data Types
Zig provides a variety of data types for working with numbers and strings. Here are some common numeric data types:
i8
,i16
,i32
,i64
: Signed integers of different sizes (8, 16, 32, 64 bits).u8
,u16
,u32
,u64
: Unsigned integers of different sizes.f32
,f64
: Floating-point numbers (32 and 64 bits).
[]const u8
: A null-terminated byte array representing a string. This is the default type for string literals.[]u8
: A byte array that may not be null-terminated.string
: A more convenient and safer string type provided by Zig's standard library.
var pi: f64 = 3.14159265359;To work with strings:
var greeting: string = "Hello, World!";
Type Inference
One of Zig's strengths is its ability to infer types when you don't explicitly specify them. This feature enhances code readability and reduces redundancy. For instance, if you assign a value to a variable during its declaration, Zig will deduce the data type:
var temperature = 22.5; // Zig infers the type as f64
However, if you declare a variable without initialization, you'll need to specify the type:
var count: i32; // Type must be explicitly provided count = 42; // Now, assign a value
Zig's type inference extends beyond variable declarations to function return types, making your code concise and expressive.
What's Next?
In this part, you've learned how to declare variables, work with numeric and string data types, and leverage Zig's type inference feature. Understanding these concepts is crucial as we continue our journey into Zig programming.
In Part 4, we'll dive into control flow in Zig, exploring conditional statements and loops, allowing you to make decisions and repeat actions in your programs. Stay tuned for more Zig insights and examples. Happy coding!