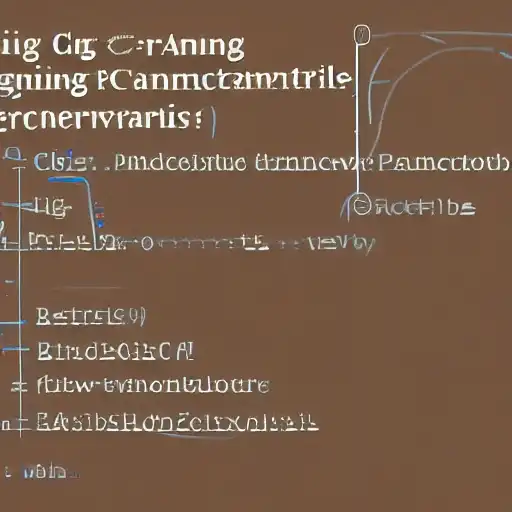
September 3rd, 2023
Welcome to the second installment of our "Getting Started with Zig on MacOS" series. In this part, we'll dive right into the practical side of Zig programming by writing your very first Zig program. We'll start with the classic "Hello, World!" example and then guide you through the process of compiling and running Zig code on your MacOS system.
Writing a "Hello, World!" Program in Zig
The "Hello, World!" program is a time-honored tradition when learning a new programming language. It's a simple yet essential example that demonstrates the basics of printing text to the screen.
Open your favorite text editor (such as Visual Studio Code, Sublime Text, or even the built-in Terminal text editor, nano) and create a new file named hello.zig
.
Now, let's write the "Hello, World!" program in Zig:
const std = @import("std"); pub fn main() void { const stdout = std.io.getStdOut().writer(); try stdout.print("Hello, World!\n"); }
Let's break down what's happening here:
- We import the
std
module, which is Zig's standard library, to access essential functionality like I/O. - Inside the
main
function, we obtain a writer for standard output (stdout) usingstd.io.getStdOut().writer()
. - We then use
try
to handle any potential errors that might occur during the printing process. - Finally, we print "Hello, World!\n" to stdout, followed by a newline character to ensure a clean output.
Compiling and Running Zig Code
Now that we've written our "Hello, World!" program, let's compile and run it:
Step 1: Compile the Program
Open your terminal and navigate to the directory where you saved hello.zig
. To compile the program, use the following command:
zig build-exe hello.zig
This command tells Zig to build an executable file named hello
from the hello.zig
source code.
Step 2: Run the Program
After successfully compiling the program, you can run it with the following command:
./hello
You should see the output:
Hello, World!
Congratulations! You've just created, compiled, and executed your first Zig program on MacOS.
What's Next?
In this part, you've taken your first steps in Zig programming by writing a "Hello, World!" program and understanding the basics of compilation and execution. In the upcoming posts, we'll delve deeper into Zig's features, data types, control flow, and more. Stay tuned for Part 3, where we'll explore variables and data types in Zig. Happy coding!