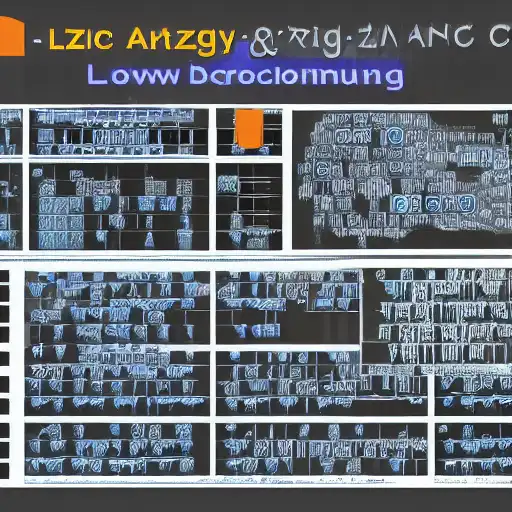
September 5th, 2023
Welcome to the fourth installment of our "Getting Started with Zig on MacOS" series. In this part, we'll explore control flow in Zig, which allows you to make decisions and repeat actions in your programs. We'll cover conditional statements such as if
, else
, and switch
, as well as looping constructs like for
and while
.
Conditional Statements
The if
Statement
The if
statement is used for conditional branching in your code. It allows you to execute a block of code if a specified condition is true. Here's a basic example:
const x: i32 = 10; if x > 5 { // Code inside this block will execute because x is greater than 5 const result = "x is greater than 5"; // You can also use result in subsequent code }
The else
Statement
You can extend the if
statement with an else
clause to provide an alternative code block to execute when the condition is false:
const y: i32 = 3; if y > 5 { // This block will not execute because y is not greater than 5 const result = "y is greater than 5"; } else { // This block will execute when the condition is false const result = "y is not greater than 5"; }
The switch
Statement
The switch
statement is used to perform different actions based on the value of an expression. It's particularly useful when you have multiple conditions to check:
const day_of_week = "Monday"; switch (day_of_week) { "Monday", "Tuesday", "Wednesday", "Thursday", "Friday" => { const result = "It's a workday!"; } "Saturday", "Sunday" => { const result = "It's the weekend!"; } else => { const result = "Invalid day"; } }
Loops
The for
Loop
Zig provides a C-style for
loop for iterating over a range of values. Here's an example:
const max = 5; for (var i: i32 = 0; i < max; i += 1) { // This block will execute 5 times with i values from 0 to 4 }
The while
Loop
The while loop repeatedly executes a block of code while a given condition is true:
const target = 10; var current = 0; while (current < target) { // This block will execute until current is no longer less than 10 current += 1; }
Control Flow Best Practices
- Use meaningful variable and function names to improve code readability.
- Avoid deeply nested control structures; consider breaking down complex logic into smaller functions.
- Use
if
,else
, andswitch
to make your code more expressive and self-documenting. - Be cautious with infinite loops; ensure there's a way to exit the loop.
- Use looping constructs that best fit your task, whether it's a fixed iteration count (
for
) or a condition-based loop (while
).
What's Next?
In this part, you've explored the essential aspects of control flow in Zig, including conditional statements (if
, else
, switch
) and loops (for
, while
). These constructs are fundamental for making your programs perform specific actions based on conditions and iterating over data.
In Part 5, we'll delve into functions and procedures in Zig, allowing you to encapsulate code into reusable modules and handle errors effectively. Stay tuned for more Zig insights and practical examples. Happy coding!