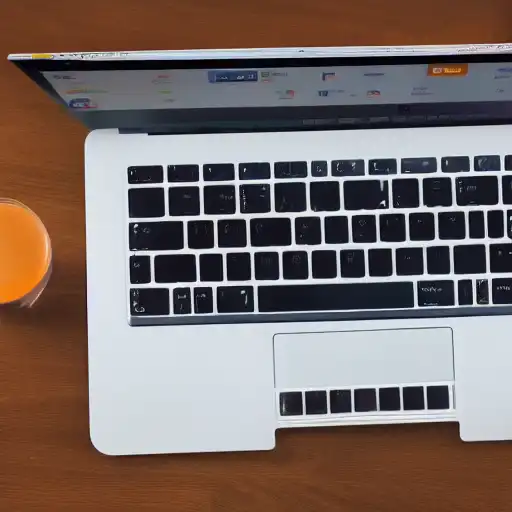
July 31st, 2023
In this post, we'll cover how to manage dependencies in Kotlin using Gradle.
Gradle is a build automation tool that is commonly used in Kotlin projects. It makes it easy to manage dependencies, build your project, and run tests.
Setting up Gradle
To use Gradle in your Kotlin project, you'll need to add the Gradle plugin to your build.gradle file:
plugins { id 'org.jetbrains.kotlin.jvm' version '1.6.3' id 'application' }
You'll also need to define the dependencies for your project. Here's an example of how to define a dependency on the Ktor server:
dependencies { implementation "io.ktor:ktor-server-netty:$ktor_version" }
You'll need to define the ktor_version
in your gradle.properties file:
ktor_version=1.6.3
Managing Dependencies
Gradle makes it easy to manage dependencies in your Kotlin project. You can define dependencies using the dependencies
block in your build.gradle file, and Gradle will automatically download the dependencies and make them available in your project.
Here's an example of how to define a dependency on the Ktor server and the MySQL driver:
dependencies { implementation "io.ktor:ktor-server-netty:$ktor_version" implementation "mysql:mysql-connector-java:8.0.27" }
This defines a dependency on the Ktor server and the MySQL driver, which will be downloaded and made available in your project.
Building and Running Your Project
Once you have your dependencies defined, you can use Gradle to build and run your project. You can use the run
task to start your application:
./gradlew run
This will start your application and listen on the port defined in your code.
You can also use the build
task to build your project:
./gradlew build
This will compile your code, run tests, and create a JAR file that you can use to run your application.
Conclusion
That's it for this post! We covered how to manage dependencies in Kotlin using Gradle. In the final post of this series, we'll wrap things up and provide some resources for further learning.