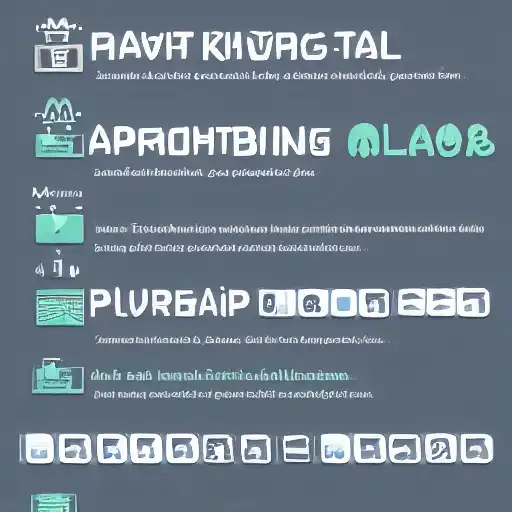
July 30th, 2023
In this post, we'll cover how to build web applications using Kotlin and the Ktor framework.
Ktor is a modern web framework for building asynchronous web applications using Kotlin. It's lightweight, flexible, and easy to use, making it a great choice for building web applications of all sizes.
Getting Started with Ktor
To get started with Ktor, you'll need to add the Ktor dependencies to your project. Here's an example of how to add the dependencies to your build.gradle file:
repositories { jcenter() } dependencies { implementation "io.ktor:ktor-server-netty:$ktor_version" implementation "io.ktor:ktor-html-builder:$ktor_version" }
You'll also need to define the Ktor version in your gradle.properties file:
ktor_version=1.6.3
Creating a Basic Application
Once you have the Ktor dependencies added to your project, you can create a basic Ktor application. Here's an example of a simple "Hello, World!" application:
import io.ktor.application.* import io.ktor.response.* import io.ktor.routing.* import io.ktor.server.engine.* import io.ktor.server.netty.* fun main() { val server = embeddedServer(Netty, port = 8080) { routing { get("/") { call.respondText("Hello, World!") } } } server.start(wait = true) }
This creates a Ktor application that listens on port 8080 and responds with "Hello, World!" when a GET request is made to the root URL.
Routing and Parameters
Ktor uses routing to handle incoming requests. You can define routes using the routing
function and handle different HTTP methods using the corresponding functions (e.g. get
, post
, put
, delete
).
Here's an example of a simple route that accepts a parameter:
routing { get("/hello/{name}") { val name = call.parameters["name"] call.respondText("Hello, $name!") } }
This route responds with "Hello, {name}!" when a GET request is made to the /hello/{name} URL, where {name} is a parameter that can be replaced with any value.
Handling Requests and Responses
Ktor uses the call
object to handle incoming requests and generate responses. You can use functions like respondText
, respondHtml
, and respondFile
to generate different types of responses.
Here's an example of responding with HTML:
routing { get("/") { call.respondHtml { head { title { +"Ktor Example" } } body { h1 { +"Hello, World!" } } } } }
This generates an HTML response with a title of "Ktor Example" and a heading of "Hello, World!".
Conclusion
That's it for this post! We covered how to build web applications using Kotlin and the Ktor framework. In the next post, we'll dive into how to manage dependencies in Kotlin using Gradle.