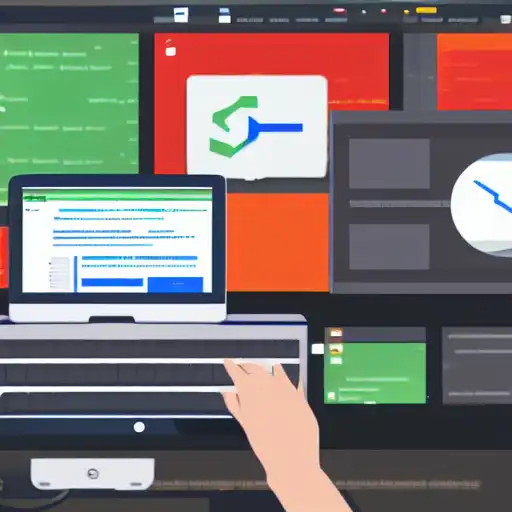
July 29th, 2023
In this post, we'll cover how to connect to databases using Kotlin.
Kotlin supports many different database libraries, including JDBC, Exposed, and Ktor. In this tutorial, we'll focus on using JDBC, which is a standard Java library for connecting to databases.
Connecting to a Database
To connect to a database using JDBC, you'll need to follow these steps:
- Add the JDBC driver to your project's dependencies. For example, if you're using MySQL, you can add the following to your build.gradle file:
dependencies { implementation 'mysql:mysql-connector-java:8.0.27' }
- Load the JDBC driver. This step is necessary to register the driver with the DriverManager so it can be used to connect to the database. Here's an example of loading the MySQL driver:
Class.forName("com.mysql.cj.jdbc.Driver")
- Open a connection to the database. This step establishes a connection to the database using the connection URL, username, and password. Here's an example:
val url = "jdbc:mysql://localhost:3306/mydatabase" val username = "myusername" val password = "mypassword" val conn = DriverManager.getConnection(url, username, password)
Executing SQL Statements
Once you have a connection to the database, you can execute SQL statements using the Statement
or PreparedStatement
classes. Here's an example of executing a SELECT
statement using a Statement
:
val stmt = conn.createStatement() val rs = stmt.executeQuery("SELECT * FROM mytable") while (rs.next()) { val id = rs.getInt("id") val name = rs.getString("name") val age = rs.getInt("age") println("id = $id, name = $name, age = $age") }
This will print out the results of the SELECT
statement.
Using Prepared Statements
Prepared statements are a way to execute SQL statements with parameters. They are more efficient than regular statements because they can be reused with different parameter values. Here's an example of using a prepared statement to insert a row into a table:
val pstmt = conn.prepareStatement("INSERT INTO mytable (name, age) VALUES (?, ?)") pstmt.setString(1, "John") pstmt.setInt(2, 30) pstmt.executeUpdate()
This will insert a row into the mytable
table with the name "John" and age 30.
Closing the Connection
Once you're done using the database connection, it's important to close it to free up resources. Here's an example of closing the connection:
conn.close()
Conclusion
That's it for this post! We covered how to connect to databases using JDBC in Kotlin. In the next post, we'll dive into how to build web applications using Kotlin and the Ktor framework.