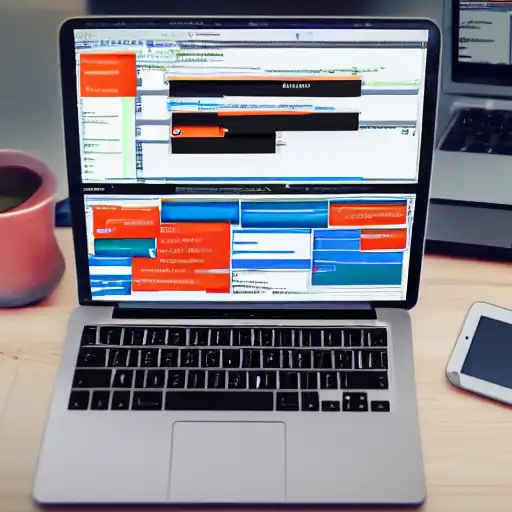
July 28th, 2023
Great, let's continue with the Kotlin tutorial series! In this post, we'll cover the basics of Kotlin syntax and some fundamental programming concepts.
Kotlin is a modern programming language that is concise, expressive, and designed to be type-safe. The syntax is very similar to Java, but Kotlin also has some unique features that make it more powerful and easier to use.
Variables and Data Types
In Kotlin, variables are declared using the keyword var
or val
. The var
keyword is used to declare mutable variables, while val
is used to declare read-only variables. Here's an example:
var x = 10 // mutable variable val y = 20 // read-only variable
Kotlin also has a number of data types, including Int
, Double
, Boolean
, and String
. Here's an example of declaring a variable with a data type:
var age: Int = 25
Functions
Functions are a key concept in programming, and Kotlin makes it easy to define and use functions. Here's an example of a simple function that takes two parameters and returns their sum:
fun sum(a: Int, b: Int): Int { return a + b }
You can call this function by passing in two integers and getting back their sum:
val result = sum(5, 10) // result is 15
Control Flow
Control flow statements are used to control the order in which statements are executed in a program. Kotlin has several control flow statements, including if
, when
, and for
loops. Here's an example of using an if
statement:
val x = 10 val y = 20 if (x > y) { println("x is greater than y") } else { println("y is greater than x") }
This will output y is greater than x
because y
is greater than x
.
Objects and Classes
Kotlin is an object-oriented language, which means that it supports classes and objects. Here's an example of defining a class in Kotlin:
class Person(val name: String, var age: Int) { fun speak() { println("My name is $name and I am $age years old.") } }
You can create an instance of this class and call its methods like this:
val person = Person("John", 30) person.speak() // Output: My name is John and I am 30 years old.
Conclusion
That's it for this post! We covered some of the basics of Kotlin syntax and fundamental programming concepts. In the next post, we'll dive into some more advanced programming concepts and show you how to connect to databases using Kotlin.