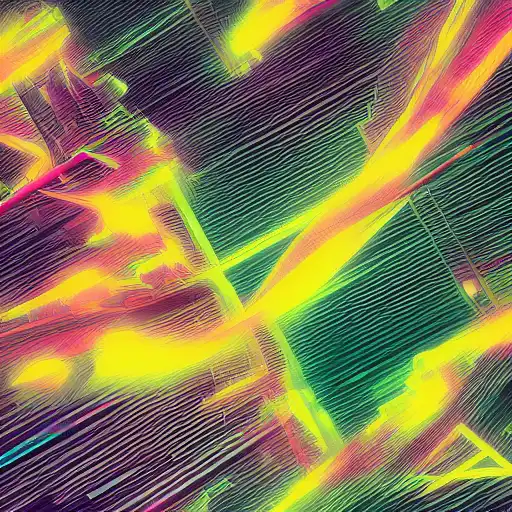
June 28th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, scientific applications, advanced numerical computing, optimization and root-finding with NLsolve.jl, statistical modeling with GLM.jl, numerical integration with QuadGK.jl, and machine learning with Flux.jl. In this post, we will focus on data manipulation in Julia, introducing the DataFrames.jl package and demonstrating how to perform various data wrangling tasks using this powerful and flexible framework.
Overview of Data Manipulation Packages in Julia
There are several data manipulation packages available in Julia, including:
- DataFrames.jl: A package for working with tabular data, providing a flexible and efficient DataFrame type, along with various functions for data manipulation, aggregation, and transformation.
- CSV.jl: A package for reading and writing CSV files, offering high-performance parsing and serialization with support for various CSV dialects and file encodings.
- SQLite.jl: A package for working with SQLite databases, providing a simple and efficient interface for querying and modifying SQLite databases using SQL statements.
In this post, we will focus on DataFrames.jl, which provides a comprehensive toolkit for data manipulation and transformation in Julia.
Getting Started with DataFrames.jl
To get started with DataFrames.jl, you first need to install the package:
import Pkg Pkg.add("DataFrames")
Now, you can create a simple DataFrame:
using DataFrames # Create a DataFrame with three columns df = DataFrame(A = 1:5, B = ["A", "B", "C", "D", "E"], C = rand(5)) # Display the DataFrame println(df)
In this example, we create a DataFrame with three columns: A, B, and C. The A column contains integer values from 1 to 5, the B column contains string values, and the C column contains random floating-point numbers.
Selecting and Filtering Data
DataFrames.jl provides various functions for selecting and filtering data:
using DataFrames # Select columns A and C selected_columns = df[:, [:A, :C]] # Filter rows where A is greater than 2 filtered_rows = df[df.A .> 2, :] # Select column B and filter rows where A is greater than 2 selected_filtered = df[df.A .> 2, :B]
In this example, we demonstrate how to select specific columns, filter rows based on a condition, and combine selection and filtering operations.
Data Transformation and Aggregation
DataFrames.jl also provides functions for data transformation and aggregation:
using DataFrames # Add a new column D with the square of A df.D = df.A .^ 2 # Compute the mean of column C mean_C = mean(df.C) # Group the DataFrame by column B and compute the sum of A and C in each group grouped = groupby(df, :B) aggregated = combine(grouped, :A => sum, :C => sum)
In this example, we demonstrate how to add a new column with a derived value, compute the mean of a column, and perform aggregation operations on grouped data.
Conclusion
In this post, we introduced data manipulation in Julia using the DataFrames.jl package. We demonstrated how to create DataFrames, select and filter data, and perform data transformation and aggregation tasks. DataFrames.jl provides a powerful and flexible framework for various applications in data analysis, data science, machine learning, and other fields.
As we continue our series on Julia, stay tuned for two more posts covering a wide range of topics. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
Keep learning, and happy coding!