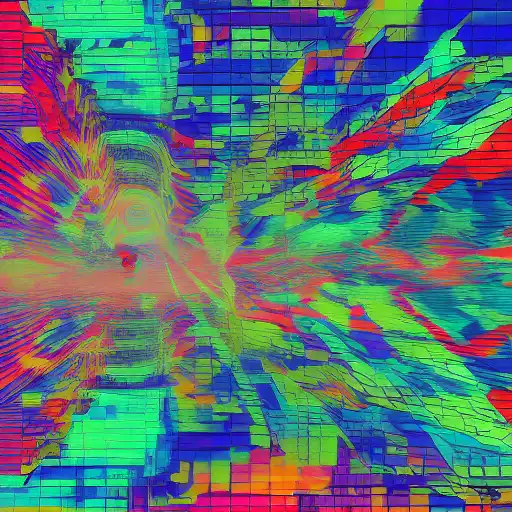
June 27th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, scientific applications, advanced numerical computing, optimization and root-finding with NLsolve.jl, statistical modeling with GLM.jl, and numerical integration with QuadGK.jl. In this post, we will focus on machine learning in Julia, introducing the Flux.jl package and demonstrating how to create and train deep learning models using this powerful and flexible framework.
Overview of Machine Learning Packages in Julia
There are several machine learning packages available in Julia, including:
- Flux.jl: A powerful and flexible deep learning framework designed from the ground up for Julia, with support for automatic differentiation, GPU acceleration, and various model architectures.
- MLJ.jl: A comprehensive toolbox for machine learning, offering a unified interface to various algorithms, tools for composing and tuning models, and support for data manipulation and preprocessing.
- ScikitLearn.jl: A wrapper around the popular Python library scikit-learn, providing a familiar interface for users coming from the Python ecosystem.
In this post, we will focus on Flux.jl, which provides an intuitive and expressive way to create and train deep learning models in Julia.
Getting Started with Flux.jl
To get started with Flux.jl, you first need to install the package:
import Pkg Pkg.add("Flux")
Now, you can use Flux to create simple deep learning models:
using Flux # Define a simple multi-layer perceptron with one hidden layer model = Chain( Dense(10, 5, relu), Dense(5, 2), softmax ) # Print the model architecture println(model)
In this example, we define a simple multi-layer perceptron with one hidden layer containing five neurons and an output layer with two neurons. We use the Chain
function to create a sequential model, and the Dense
function to create fully connected layers. The activation functions are specified using the relu
and softmax
functions.
Training a Deep Learning Model
To train a deep learning model with Flux, you need to define a loss function, an optimizer, and a dataset:
using Flux, Random # Generate a synthetic dataset X = rand(10, 100) Y = onehotbatch(rand(1:2, 100), 1:2) # Define the loss function loss(x, y) = Flux.Losses.crossentropy(model(x), y) # Define the optimizer optimizer = ADAM(0.001) # Train the model Flux.train!(loss, params(model), [(X, Y)], optimizer)
In this example, we generate a synthetic dataset with 10-dimensional input features and two output classes. We define the loss function using the crossentropy
function from Flux's Losses
submodule. The optimizer is defined using the ADAM
function with a learning rate of 0.001. The train!
function is used to update the model's parameters during training.
Monitoring Training Progress
To monitor the progress of training, you can use Flux's built-in callback system:
using Flux # Define the callback function callback() = @info("Loss: $(loss(X, Y))") # Train the model with the callback Flux.train!(loss, params(model), [(X, Y)], optimizer, cb=throttle(callback, 10))
In this example, we define a callback function that prints the current value of the loss function. The `@info` macro is used to display the information in a formatted manner. The `throttle` function is used to limit the frequency of the callback execution to once every 10 seconds.
Evaluating and Saving the Trained Model
Once the model is trained, you can evaluate its performance on a test dataset and save the trained model for future use:
using Flux, BSON # Generate a test dataset X_test = rand(10, 50) Y_test = onehotbatch(rand(1:2, 50), 1:2) # Compute the accuracy of the model on the test dataset accuracy(x, y) = mean(onecold(model(x)) .== onecold(y)) println("Test accuracy: $(accuracy(X_test, Y_test))") # Save the trained model to a file BSON.@save "trained_model.bson" model
In this example, we generate a test dataset and compute the accuracy of the trained model using the accuracy
function. The onecold
function is used to convert the model's output probabilities into class labels. Finally, we save the trained model to a BSON file using the BSON package and the @save
macro.
Conclusion
In this post, we introduced machine learning in Julia using the Flux.jl package. We demonstrated how to create and train deep learning models using an intuitive and expressive syntax, with support for automatic differentiation, GPU acceleration, and various model architectures. Flux.jl provides a powerful and flexible framework for various applications in machine learning, data analysis, and other fields.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from parallel processing and distributed computing to high-performance computing and scientific applications. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into advanced numerical computing, discussing topics such as data manipulation with DataFrames.jl, optimization with JuMP.jl, and graph algorithms with LightGraphs.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!