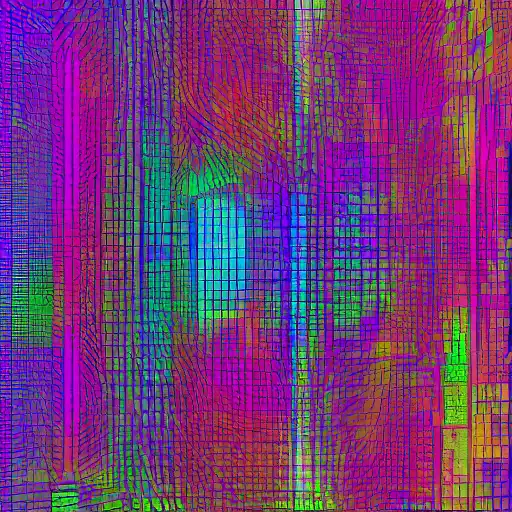
June 26th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, scientific applications, advanced numerical computing, optimization and root-finding with NLsolve.jl, and statistical modeling with GLM.jl. In this post, we will focus on numerical integration in Julia, introducing the QuadGK.jl package and demonstrating how to perform numerical integration of single and multiple variables functions using this powerful and flexible framework.
Overview of Numerical Integration Packages in Julia
There are several numerical integration packages available in Julia, including:
- QuadGK.jl: A package for one-dimensional adaptive Gauss-Kronrod quadrature, which is a widely used method for numerical integration.
- Cubature.jl: A package for multi-dimensional adaptive quadrature using various algorithms, such as the Genz-Malik and Berntsen-Espelid methods.
- HCubature.jl: A package for multi-dimensional adaptive quadrature using the same underlying algorithm as Cubature.jl but with a different implementation that emphasizes performance.
In this post, we will focus on QuadGK.jl, which provides efficient and accurate one-dimensional numerical integration using the adaptive Gauss-Kronrod quadrature method.
Getting Started with QuadGK.jl
To get started with QuadGK.jl, you first need to install the package:
import Pkg Pkg.add("QuadGK")
Now, you can use the quadgk
function to perform numerical integration of a one-dimensional function:
using QuadGK # Define the function to be integrated f(x) = x^2 # Integrate the function from 0 to 1 result, error = quadgk(f, 0, 1) # Print the result and the estimated error println("Result: ", result) println("Estimated error: ", error)
In this example, we define a simple function f(x) = x^2
and integrate it over the interval [0, 1]
. The quadgk
function returns the result of the integration and an estimate of the error.
Numerical Integration with Singularities
QuadGK.jl can also handle functions with singularities by specifying the locations of the singularities as additional arguments:
using QuadGK # Define a function with a singularity at x = 0 f(x) = 1 / sqrt(x) # Integrate the function from 0 to 1, specifying the singularity at x = 0 result, error = quadgk(f, 0, 1, singularities=[0]) # Print the result and the estimated error println("Result: ", result) println("Estimated error: ", error)
In this example, we define a function f(x) = 1 / sqrt(x)
with a singularity at x = 0
. We integrate the function over the interval [0, 1]
, specifying the location of the singularity as an additional argument to the quadgk
function. The result and estimated error are printed as before.
Multi-dimensional Integration with Cubature.jl
For multi-dimensional integration, you can use the Cubature.jl package:
import Pkg Pkg.add("Cubature") using Cubature # Define a two-dimensional function to be integrated f(x) = x[1]^2 + x[2]^2 # Integrate the function over the domain [0, 1] x [0, 1] result, error = hcubature(f, [0, 0], [1, 1]) # Print the result and the estimated error println("Result: ", result) println("Estimated error: ", error)
In this example, we define a two-dimensional function f(x) = x[1]^2 + x[2]^2
and integrate it over the domain [0, 1] x [0, 1]
. We use the hcubature
function from the Cubature.jl package, which performs adaptive multi-dimensional integration. The result and estimated error are printed as before.
Conclusion
In this post, we introduced numerical integration in Julia using the QuadGK.jl and Cubature.jl packages. We demonstrated how to perform one-dimensional numerical integration using the adaptive Gauss-Kronrod quadrature method and multi-dimensional integration using adaptive quadrature algorithms. These packages provide efficient and accurate numerical integration tools for various applications in scientific computing, engineering, data analysis, and other fields.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from parallel processing and distributed computing to high-performance computing and scientific applications. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into advanced numerical computing, discussing topics such as machine learning with Flux.jl, data manipulation with DataFrames.jl, and optimization with JuMP.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!