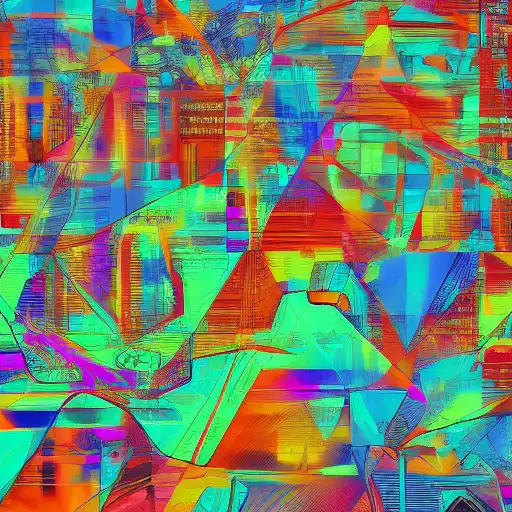
June 25th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, scientific applications, advanced numerical computing, optimization and root-finding with NLsolve.jl. In this post, we will focus on statistical modeling in Julia, introducing the GLM.jl package and demonstrating how to perform linear regression, logistic regression, and other generalized linear models using this powerful and flexible framework.
Overview of Statistical Modeling Packages in Julia
There are several statistical modeling packages available in Julia, including:
- GLM.jl: A package for fitting generalized linear models, including linear regression, logistic regression, and Poisson regression.
- MixedModels.jl: A package for fitting linear mixed-effects models, which are useful for modeling clustered or longitudinal data.
- StatsModels.jl: A package for specifying and fitting statistical models using formulas, which provides a convenient way to specify complex models.
In this post, we will focus on GLM.jl, which provides a wide range of generalized linear models and is widely used for various applications in data analysis, econometrics, and other fields.
Getting Started with GLM.jl
To get started with GLM.jl, you first need to install the package:
import Pkg Pkg.add("GLM")
Now, you can use the lm
and glm
functions to fit linear regression and generalized linear models, respectively:
using GLM, DataFrames # Load the dataset data = DataFrame(X1=randn(100), X2=randn(100), X3=randn(100)) data.Y = 2 * data.X1 - 3 * data.X2 + 4 * data.X3 + randn(100) # Fit a linear regression model model = lm(@formula(Y ~ X1 + X2 + X3), data) # Print the model summary println(model)
In this example, we create a synthetic dataset with three predictor variables X1
, X2
, and X3
, and a response variable Y
. We fit a linear regression model to the data using the lm
function and the @formula
macro, which specifies the model in a convenient, R-like syntax. The model summary is printed, showing the estimated coefficients, standard errors, and other statistics.
Fitting Generalized Linear Models
In addition to linear regression, GLM.jl also supports other generalized linear models, such as logistic regression and Poisson regression. In this example, we demonstrate how to fit a logistic regression model:
using GLM, DataFrames # Load the dataset data = DataFrame(X1=randn(100), X2=randn(100), X3=randn(100)) data.Y = ifelse.(2 * data.X1 - 3 * data.X2 + 4 * data.X3 .+ randn(100) .> 0, 1, 0) # Fit a logistic regression model model = glm(@formula(Y ~ X1 + X2 + X3), data, Binomial(), LogitLink()) # Print the model summary println(model)
In this example, we use the same synthetic dataset as before, but we transform the response variable Y
into a binary outcome based on whether the linear predictor is greater than zero. We fit a logistic regression model to the data using the glm
function, specifying the Binomial
distribution and the LogitLink
function to model the relationship between the predictors and the binary response variable. The model summary is printed, showing the estimated coefficients, standard errors, and other statistics.
Model Diagnostics and Goodness-of-Fit
After fitting a generalized linear model, it is important to assess the model's diagnostics and goodness-of-fit. GLM.jl provides various tools for this purpose, such as residual plots, deviance, and AIC. In this example, we demonstrate how to compute these statistics for a linear regression model:
using GLM, DataFrames # Fit a linear regression model data = DataFrame(X1=randn(100), X2=randn(100), X3=randn(100)) data.Y = 2 * data.X1 - 3 * data.X2 + 4 * data.X3 + randn(100) model = lm(@formula(Y ~ X1 + X2 + X3), data) # Compute residuals residuals = residuals(model) # Compute deviance deviance = deviance(model) # Compute AIC aic = aic(model) # Print the results println("Residuals: ", residuals) println("Deviance: ", deviance) println("AIC: ", aic)
In this example, we fit a linear regression model to the synthetic dataset and compute various diagnostic and goodness-of-fit statistics. The residuals
function computes the model's residuals, the deviance
function computes the model's deviance, and the aic
function computes the model's Akaike Information Criterion (AIC), which is a measure of model fit that balances goodness-of-fit and model complexity.
Conclusion
In this post, we introduced statistical modeling in Julia using the GLM.jl package. We demonstrated how to fit linear regression, logistic regression, and other generalized linear models using a convenient formula syntax. GLM.jl provides a wide range of models and tools for model diagnostics and goodness-of-fit, making it a powerful and flexible framework for various applications in data analysis, econometrics, and other fields.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from parallel processing and distributed computing to high-performance computing and scientific applications. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into advanced numerical computing, discussing topics such as numerical integration with QuadGK.jl, machine learning with Flux.jl, and data manipulation with DataFrames.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!