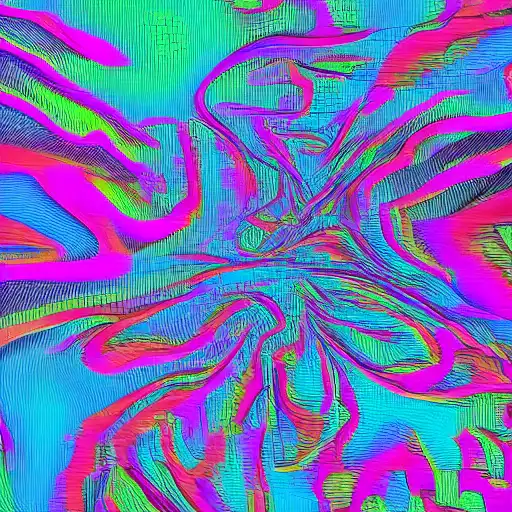
June 24th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, scientific applications, and advanced numerical computing. In this post, we will focus on optimization and root-finding in Julia, introducing the NLsolve.jl package and demonstrating how to solve nonlinear equations and optimization problems using this powerful and flexible framework.
Overview of Optimization and Root-Finding Packages in Julia
There are several optimization and root-finding packages available in Julia, including:
- NLsolve.jl: A package for solving nonlinear equations and systems of nonlinear equations.
- Optim.jl: A package for optimization problems, including unconstrained and constrained optimization, as well as linear and quadratic programming.
- JuMP.jl: A modeling language for mathematical optimization, which we have covered in a previous post.
In this post, we will focus on NLsolve.jl, which provides efficient algorithms for solving nonlinear equations and systems of nonlinear equations.
Getting Started with NLsolve.jl
To get started with NLsolve.jl, you first need to install the package:
import Pkg Pkg.add("NLsolve")
Now, you can use the nlsolve
function to solve a system of nonlinear equations:
using NLsolve # Define the system of equations function f!(F, x) F[1] = x[1]^2 + x[2]^2 - 1 F[2] = x[1] * x[2] - 0.5 end # Define the initial guess x0 = [0.5, 0.5] # Solve the system of equations solution = nlsolve(f!, x0) # Extract the solution x = solution.zero
In this example, we solve the system of nonlinear equations x₁² + x₂² = 1
and x₁ * x₂ = 0.5
. The f!
function defines the system of equations, and the nlsolve
function solves the system using a default algorithm. The resulting solution can be extracted using the zero
field of the solution object.
Solving Optimization Problems with NLsolve.jl
Although NLsolve.jl is primarily designed for solving nonlinear equations, it can also be used to solve optimization problems by reformulating them as root-finding problems. In this example, we will demonstrate how to solve an unconstrained optimization problem:
using NLsolve # Define the objective function and its gradient function f(x) return (x[1] - 1)^2 + (x[2] - 2)^2 end function g!(G, x) G[1] = 2 * (x[1] - 1) G[2] = 2 * (x[2] - 2) end # Define the initial guess x0 = [0.0, 0.0] # Solve the optimization problem solution = nlsolve(g!, x0) # Extract the solution x_opt = solution.zero
In this example, we solve the unconstrained optimization problem minimize (x₁ - 1)² + (x₂ - 2)²
. The f
function defines the objective function, and the g!
function defines its gradient. The optimization problem is reformulated as a root-finding problem by finding the zeros of the gradient. The nlsolve
function is then used to solve the root-finding problem, and the resulting solution is the optimal point for the original optimization problem.
Customizing the Solver
NLsolve.jl provides several options for customizing the solver, such as choosing a different algorithm or adjusting the convergence tolerance. In this example, we demonstrate how to customize the solver using the method
and xtol
options:
using NLsolve # Define the system of equations function f!(F, x) F[1] = x[1]^2 + x[2]^2 - 1 F[2] = x[1] * x[2] - 0.5 end # Define the initial guess x0 = [0.5, 0.5] # Customize the solver solver_options = NLsolve.NewtonTrustRegion(; xtol=1e-10) # Solve the system of equations with custom options solution = nlsolve(f!, x0, method=solver_options) # Extract the solution x = solution.zero
In this example, we customize the solver by using the Newton trust region algorithm with a convergence tolerance of 1e-10
. The NLsolve.NewtonTrustRegion
function creates a solver with the specified options, which is then passed to the nlsolve
function using the method
argument.
Conclusion
In this post, we introduced optimization and root-finding in Julia using the NLsolve.jl package. We demonstrated how to solve nonlinear equations and systems of nonlinear equations, as well as how to solve optimization problems by reformulating them as root-finding problems. NLsolve.jl provides efficient algorithms for solving a wide range of problems in scientific computing, engineering, data science, and other disciplines that require nonlinear equation solving and optimization.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from parallel processing and distributed computing to high-performance computing and scientific applications. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into advanced numerical computing, discussing topics such as statistical modeling with GLM.jl, numerical integration with QuadGK.jl, and machine learning with Flux.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!