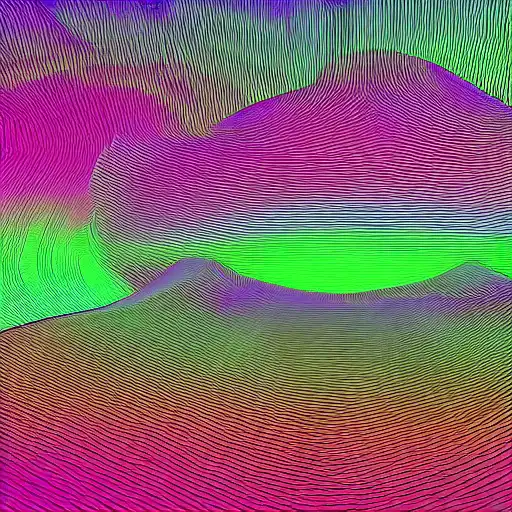
June 23rd, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, and scientific applications. In this post, we will focus on advanced numerical computing in Julia, introducing the LinearAlgebra.jl package and demonstrating how to perform various linear algebra operations using this powerful and flexible framework.
Overview of Linear Algebra Packages in Julia
There are several linear algebra packages available in Julia, including:
- LinearAlgebra.jl: A standard library for linear algebra in Julia that provides various operations such as matrix multiplication, matrix factorization, and eigenvalue decomposition.
- IterativeSolvers.jl: A package for iterative algorithms for solving linear systems and eigenvalue problems.
- SparseArrays.jl: A standard library for working with sparse matrices in Julia, which can be used in conjunction with LinearAlgebra.jl to perform linear algebra operations on sparse matrices efficiently.
In this post, we will focus on LinearAlgebra.jl, which is part of the standard library and is loaded by default in every Julia session. It provides a wide range of linear algebra functions and allows you to perform matrix operations efficiently using the high-performance capabilities of Julia.
Basic Linear Algebra Operations in Julia
To get started with LinearAlgebra.jl, you first need to install the package:
import Pkg Pkg.add("LinearAlgebra")
Now, you can perform various linear algebra operations, such as matrix multiplication, matrix inversion, and matrix factorization:
# Create two matrices A = [1 2; 3 4] B = [2 1; 4 3] # Matrix multiplication C = A * B # Matrix inversion A_inv = inv(A) # Matrix factorization (LU decomposition) F = lu(A) # Extract the L and U matrices L = F.L U = F.U # Solve a linear system x = A \ [5; 6]
In this example, we perform basic linear algebra operations on two 2x2 matrices A
and B
. We multiply the matrices, compute the inverse of A
, perform an LU decomposition of A
, and solve a linear system Ax = b
with b = [5; 6]
. The inv
function computes the matrix inverse, the lu
function performs the LU decomposition, and the backslash operator \
solves the linear system.
Working with Sparse Matrices
In addition to dense matrices, LinearAlgebra.jl can also work with sparse matrices efficiently using the SparseArrays.jl package:
using SparseArrays # Create a sparse matrix S = sparse([1, 2, 3], [1, 2, 3], [1.0, 2.0, 3.0]) # Convert the sparse matrix to a dense matrix D = Matrix(S) # Perform matrix-vector multiplication v = [1; 2; 3] result = S * v
In this example, we create a sparse matrix S
, convert it to a dense matrix D
, and perform matrix-vector multiplication with a vector v
. The sparse
function creates a sparse matrix from row, column, and value vectors, and the Matrix
function converts a sparse matrix to a dense matrix.
Conclusion
In this post, we introduced advanced numerical computing in Julia using the LinearAlgebra.jl package. We demonstrated how to perform various linear algebra operations, such as matrix multiplication, matrix inversion, and matrix factorization, using this powerful and flexible framework. Furthermore, we showed how to work with sparse matrices efficiently using the SparseArrays.jl package.
With LinearAlgebra.jl, you can efficiently perform linear algebra operations on dense and sparse matrices, leveraging the high-performance capabilities of Julia. This package is essential for various applications in scientific computing, data science, engineering, and other disciplines that require advanced numerical computing.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from parallel processing and distributed computing to high-performance computing and scientific applications. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into advanced numerical computing, discussing topics such as optimization and root-finding with NLsolve.jl, statistical modeling with GLM.jl, and numerical integration with QuadGK.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!