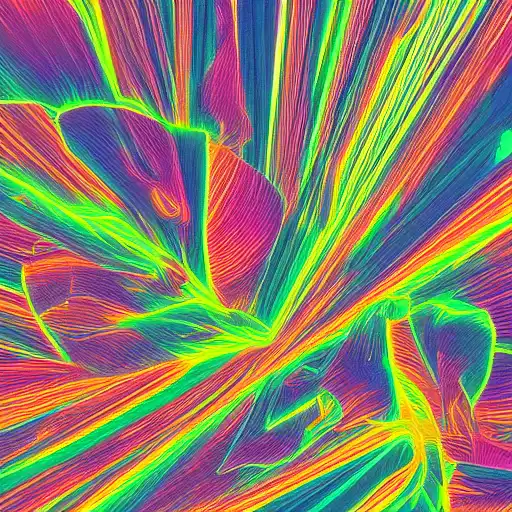
June 22nd, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, and mathematical optimization. In this post, we will focus on scientific applications in Julia, introducing the DifferentialEquations.jl package and demonstrating how to solve ordinary, partial, and stochastic differential equations using this powerful and flexible framework.
Overview of Differential Equations Packages in Julia
There are several differential equation packages available in Julia, including:
- DifferentialEquations.jl: A comprehensive suite for numerically solving various types of differential equations, including ordinary, partial, stochastic, and delay differential equations.
- OrdinaryDiffEq.jl: A package for solving ordinary differential equations (ODEs) that is part of the DifferentialEquations.jl ecosystem.
- BoundaryValueDiffEq.jl: A package for solving boundary value problems (BVPs) for ordinary and partial differential equations, which is also part of the DifferentialEquations.jl ecosystem.
In this post, we will focus on DifferentialEquations.jl, which provides a high-level interface for solving a wide range of differential equation problems.
Getting Started with DifferentialEquations.jl
To get started with DifferentialEquations.jl, you first need to install the package:
import Pkg Pkg.add("DifferentialEquations")
Now, you can use the ODEProblem
, solve
, and plot
functions to define, solve, and visualize an ordinary differential equation (ODE):
using DifferentialEquations, Plots # Define the ODE function f(u, p, t) return -u end # Define the initial condition u0 = 1.0 # Define the time span tspan = (0.0, 5.0) # Create the ODE problem problem = ODEProblem(f, u0, tspan) # Solve the ODE solution = solve(problem) # Plot the solution plot(solution)
In this example, we solve the first-order ODE du/dt = -u
with the initial condition u(0) = 1
. The ODEProblem
function creates an ODE problem instance, which is then solved using the solve
function. The resulting solution can be visualized using the plot
function from the Plots package.
Solving Partial Differential Equations (PDEs)
DifferentialEquations.jl also supports solving partial differential equations (PDEs) using a variety of methods. To solve a PDE, you first need to transform it into a system of ODEs or a system of stochastic differential equations (SDEs). In this example, we will demonstrate how to solve the heat equation, a widely-studied PDE in physics and engineering:
using DifferentialEquations, Plots # Define the PDE function heat_equation(du, u, p, t) α, Δx = p for i in 2:length(u) - 1 du[i] = α * (u[i - 1] - 2 * u[i] + u[i + 1]) / Δx^2 end du[1] = du[end] = 0 end # Define the initial condition u0 = [sin(2 * π * x) for x in 0:0.01:1] # Define the time span tspan = (0.0, 0.1) # Define the PDE parameters α = 0.1 Δx = 0.01 # Create the PDE problem problem = ODEProblem(heat_equation, u0, tspan, (α, Δx)) # Solve the PDE solution = solve(problem) # Plot the solution heatmap(solution)
In this example, we solve the heat equation ∂u/∂t = α * ∂²u/∂x²
using an explicit finite difference method. The heat_equation
function defines the PDE, which is transformed into a system of ODEs using a spatial discretization with step size Δx
. The initial condition is given by a sine wave, and the boundary conditions are set to zero. The resulting ODE problem is then solved using the solve
function, and the solution is visualized as a heatmap.
Solving Stochastic Differential Equations (SDEs)
DifferentialEquations.jl also provides support for solving stochastic differential equations (SDEs). In this example, we will demonstrate how to solve a simple SDE with additive noise:
using DifferentialEquations, Plots, Random # Define the SDE function f(u, p, t) return -u end function g(u, p, t) return 0.1 end # Define the initial condition u0 = 1.0 # Define the time span tspan = (0.0, 5.0) # Create the SDE problem problem = SDEProblem(f, g, u0, tspan) # Set a random seed for reproducibility Random.seed!(42) # Solve the SDE solution = solve(problem) # Plot the solution plot(solution)
In this example, we solve the first-order SDE du/dt = -u + 0.1 * dW(t)
with the initial condition u(0) = 1
. The SDEProblem
function creates an SDE problem instance, which is then solved using the solve
function. The resulting solution can be visualized using the plot
function from the Plots package.
Conclusion
In this post, we introduced scientific applications in Julia using the DifferentialEquations.jl package. We demonstrated how to solve ordinary, partial, and stochastic differential equations using this powerful and flexible framework. With DifferentialEquations.jl, you can efficiently model and solve a wide range of problems in physics, engineering, biology, and other disciplines that involve differential equations, leveraging the high-performance capabilities of Julia.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from advanced numerical computing and parallel processing to distributed computing and high-performance computing. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into scientific applications, discussing topics such as numerical linear algebra with LinearAlgebra.jl, optimization and root-finding with NLsolve.jl, and statistical modeling with GLM.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!