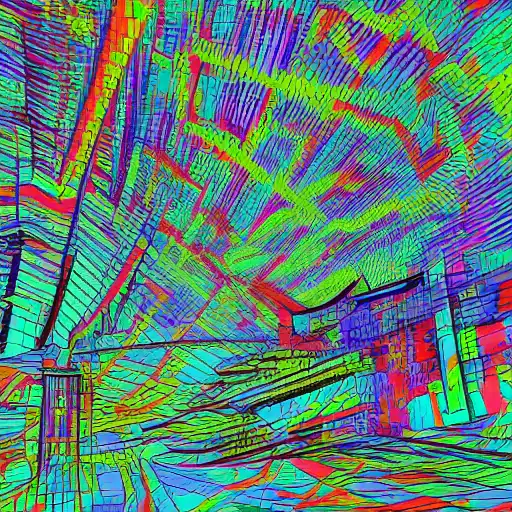
June 21st, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, and deep learning. In this post, we will focus on mathematical optimization in Julia, introducing the JuMP.jl package and demonstrating how to formulate and solve optimization problems using this powerful and flexible framework.
Overview of Mathematical Optimization Packages in Julia
There are several mathematical optimization packages available in Julia, including:
- JuMP.jl: A high-level modeling language for mathematical optimization that allows you to easily formulate and solve linear, mixed-integer, quadratic, and nonlinear optimization problems.
- Convex.jl: A package for disciplined convex programming, which is a subset of mathematical optimization that focuses on convex optimization problems.
- Optim.jl: A package for general-purpose optimization that provides algorithms for unconstrained and constrained optimization, as well as line search and trust region methods.
In this post, we will focus on JuMP.jl, which is one of the most popular and widely used mathematical optimization libraries in the Julia ecosystem.
Getting Started with JuMP.jl
To get started with JuMP.jl, you first need to install the package:
import Pkg Pkg.add("JuMP")
In addition to JuMP.jl, you will also need to install an optimization solver that is compatible with JuMP.jl. Some popular solvers include:
- GLPK.jl for linear programming and mixed-integer linear programming.
- Ipopt.jl for nonlinear programming.
- Gurobi.jl for linear, quadratic, and mixed-integer programming (requires a Gurobi license).
For this post, we will use GLPK.jl as the optimization solver:
Pkg.add("GLPK")
Now, you can use the Model
function to create an optimization model and the @variable
, @constraint
, and @objective
macros to define variables, constraints, and the objective function:
using JuMP, GLPK # Create an optimization model model = Model(GLPK.Optimizer) # Define variables @variable(model, x >= 0) @variable(model, y >= 0) # Define constraints @constraint(model, x + y <= 1) # Define the objective function @objective(model, Max, x + 2 * y)
In this example, we create a linear programming model with two variables x
and y
, subject to a single constraint x + y <= 1
. The objective is to maximize the expression x + 2 * y
.
Solving the Optimization Problem
To solve the optimization problem, you can use the optimize!
function:
optimize!(model)
After solving the problem, you can use the objective_value
and value
functions to obtain the optimal objective value and variable values:
# Get the optimal objective value optimal_objective = objective_value(model) # Get the optimal variable values optimal_x = value(x) optimal_y = value(y) println("Optimal objective value: ", optimal_objective) println("Optimal x: ", optimal_x) println("Optimal y: ", optimal_y)
Conclusion
In this post, we introduced mathematical optimization in Julia using the JuMP.jl package. We demonstrated how to formulate and solve optimization problems using this powerful and flexible framework. With JuMP.jl, you can easily model and solve a wide range of optimization problems, from linear and mixed-integer programming to quadratic and nonlinear programming, leveraging the high-performance capabilities of Julia.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from scientific applications and advanced numerical computing to parallel processing and distributed computing. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In upcoming posts, we will delve deeper into optimization techniques, discussing topics such as constraint programming with ConstraintSolver.jl, global optimization with BlackBoxOptim.jl, and multi-objective optimization with MOEAFramework.jl. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!