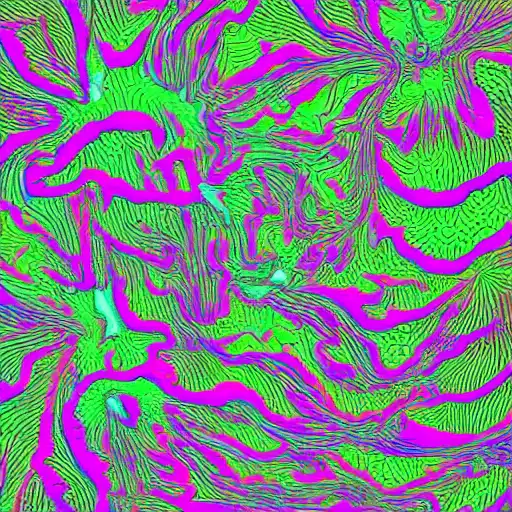
June 20th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, and time series forecasting. In this post, we will focus on deep learning in Julia, introducing the Flux.jl package and demonstrating how to build and train neural networks using this powerful framework.
Overview of Deep Learning Packages in Julia
There are several deep learning packages available in Julia, including:
Flux.jl
: A flexible and high-performance deep learning library that provides the necessary building blocks for creating, training, and evaluating neural networks.Knet.jl
: A deep learning framework that emphasizes the use of custom and efficient GPU kernels for common neural network operations.ONNX.jl
: A package that allows you to import and export neural networks using the Open Neural Network Exchange (ONNX) format, making it easier to interoperate with other deep learning frameworks, such as TensorFlow and PyTorch.
In this post, we will focus on Flux.jl, which is one of the most popular and widely used deep learning libraries in the Julia ecosystem.
Getting Started with Flux.jl
To get started with Flux.jl, you first need to install the package:
import Pkg Pkg.add("Flux")
Now, you can use the Chain
function to create a simple feedforward neural network:
using Flux model = Chain( Dense(10, 5, relu), Dense(5, 2), softmax )
The Chain
function composes multiple layers into a single neural network, where the output of one layer is passed as input to the next layer. In this example, we create a network with two Dense
layers followed by a softmax
activation function, which is commonly used for multi-class classification tasks.
Training a Neural Network with Flux.jl
To train a neural network with Flux.jl, you first need to define a loss function, an optimization algorithm, and a dataset:
using Flux: crossentropy, ADAM # Define a dummy dataset X = rand(10, 100) Y = rand(0:1, 2, 100) # Define the loss function loss(x, y) = crossentropy(model(x), y) # Define the optimization algorithm opt = ADAM()
In this example, we use the crossentropy
loss function for multi-class classification and the ADAM
optimization algorithm, which is a popular choice for training deep neural networks.
Next, you can use the train!
function to train the neural network:
using Flux: train! # Train the neural network for 10 epochs for epoch in 1:10 train!(loss, Flux.params(model), [(X, Y)], opt) end
The train!
function updates the parameters of the neural network using the specified optimization algorithm and loss function. In this example, we train the neural network for 10 epochs using the provided dataset.
Evaluating the Neural Network
After training the neural network, you can use the accuracy function to evaluate its performance on a test dataset:
using Flux: onecold # Define a dummy test dataset X_test = rand(10, 20) Y_test = rand(0:1, 2, 20) # Define the accuracy function accuracy(x, y) = mean(onecold(model(x)) .== onecold(y)) # Compute the accuracy on the test dataset test_accuracy = accuracy(X_test, Y_test) println("Test accuracy: ", test_accuracy)
The accuracy
function computes the proportion of correct predictions made by the neural network on the test dataset. In this example, we use the onecold
function to convert the output probabilities of the neural network into class labels and compare them to the true labels.
Conclusion
In this post, we introduced deep learning in Julia using the Flux.jl package. We demonstrated how to build, train, and evaluate neural networks using this powerful and flexible framework. With Flux.jl, you can create complex deep learning models and train them efficiently using the high-performance capabilities of Julia.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from mathematical optimization and scientific applications to advanced numerical computing and parallel processing. We will explore various packages and techniques, equipping you with the knowledge and skills required to tackle complex problems in your domain.
Keep learning, and happy coding!