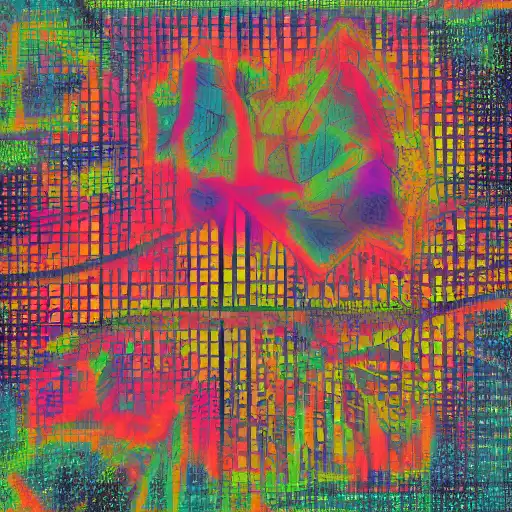
June 19th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, and data visualization. In this post, we will focus on time series forecasting in Julia, exploring how to analyze and forecast time series data using the TimeSeries.jl and ARCHModels.jl packages.
Overview of Time Series Packages in Julia
There are several packages available in Julia for working with time series data, including:
- TimeSeries.jl: A package for working with time series data, providing functionality for handling, transforming, and summarizing time series data.
- ARCHModels.jl: A package for estimating and forecasting volatility in time series data using autoregressive conditional heteroskedasticity (ARCH) models.
- StateSpaceModels.jl: A package for state space modeling and the Kalman filter, which can be used for time series forecasting.
In this post, we will focus on using TimeSeries.jl for handling time series data and ARCHModels.jl for volatility forecasting.
Working with Time Series Data using TimeSeries.jl
To get started with TimeSeries.jl, you first need to install the package:
import Pkg Pkg.add("TimeSeries")
Now, you can create a TimeArray
object to represent time series data:
using TimeSeries dates = Date(2020, 1, 1):Date(2020, 12, 31) values = rand(length(dates)) time_series = TimeArray(dates, values)
The TimeArray
object provides various functions for handling time series data, such as:
timestamp
: Get the time indices of the time series.values
: Get the values of the time series.lag
: Compute the lagged values of the time series.lead
: Compute the lead values of the time series.moving
: Compute the moving average, median, or other summary statistics of the time series.
For example, to compute the 5-day moving average of a time series, you can use the following code:
moving_average = moving(time_series, 5, mean)
Volatility Forecasting using ARCHModels.jl
To forecast volatility in time series data using ARCH models, you can use the ARCHModels.jl package. First, let's install the package:
import Pkg Pkg.add("ARCHModels")
Now, you can estimate an ARCH or GARCH model using the fit
function:
using ARCHModels # Estimate a GARCH(1, 1) model garch_model = fit(GARCH{1, 1}, values)
Once you have estimated the model, you can use the forecast
function to forecast volatility:
# Forecast volatility for the next 10 days volatility_forecast = forecast(garch_model, 10)
Conclusion
In this post, we introduced time series forecasting in Julia using the TimeSeries.jl and ARCHModels.jl packages. We demonstrated how to handle time series data using TimeSeries.jl and how to forecast volatility using ARCHModels.jl. With these tools, you can efficiently analyze and forecast time series data, enabling you to make better decisions based on historical patterns and trends.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from statistical analysis and machine learning to advanced numerical computing and scientific applications. We will delve into various aspects of the language and its packages, equipping you with the knowledge and skills required to tackle complex problems in your domain.
In the upcoming posts, we will explore other popular packages and techniques, such as the use of the Flux.jl package for deep learning, the JuMP.jl package for mathematical optimization, and the DifferentialEquations.jl package for solving differential equations. These topics will further enhance your understanding of Julia and its capabilities, enabling you to become a proficient Julia programmer.
Keep learning, and happy coding!