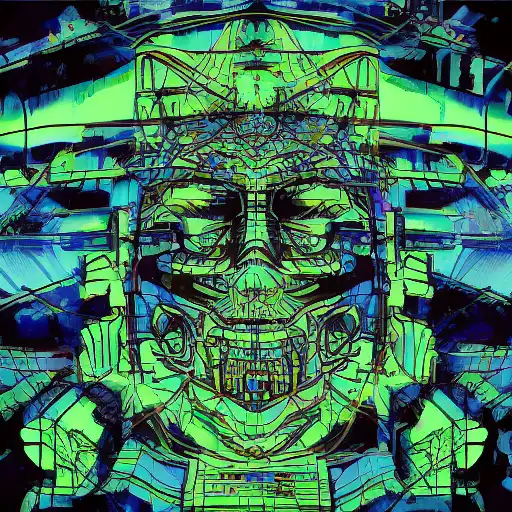
June 18th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, and web scraping. In this post, we will focus on data visualization in Julia, exploring how to create various types of plots using the Plots.jl and Makie.jl packages.
Overview of Data Visualization Packages in Julia
There are several data visualization packages available in Julia, including:
- Plots.jl: A high-level data visualization package that provides a unified interface for multiple backends, including GR, Plotly, PyPlot, and others.
- Makie.jl: A high-performance, flexible, and interactive data visualization package that supports various output formats, including SVG, PNG, and WebGL.
- Gadfly.jl: A package for creating static, publication-quality graphics using a concise and expressive syntax, inspired by the R package ggplot2.
In this post, we will focus on using Plots.jl for creating various types of plots and Makie.jl for creating interactive and high-performance visualizations.
Creating Plots with Plots.jl
To get started with Plots.jl, you first need to install the package:
import Pkg Pkg.add("Plots")
Now, you can use the plot
function to create simple plots. For example, to create a line plot of a sine function, you can use the following code:
using Plots x = 0:0.1:2π y = sin.(x) plot(x, y, label="sin(x)")
Plots.jl supports various types of plots, including line plots, scatter plots, bar plots, histograms, and more. You can customize the appearance of the plots by specifying various attributes, such as colors, markers, and line styles.
For example, to create a scatter plot with custom markers and colors, you can use the following code:
x = 1:10 y = rand(10) scatter(x, y, markershape=:diamond, markercolor=:red, label="Random data")
Creating Subplots with Plots.jl
You can create subplots using the plot
function by specifying the layout and passing multiple series of data. For example, to create a 2x2 grid of subplots with different types of plots, you can use the following code:
x = 1:10 y1 = rand(10) y2 = rand(10) y3 = rand(10) y4 = rand(10) p1 = plot(x, y1, label="Line plot") p2 = scatter(x, y2, label="Scatter plot") p3 = bar(x, y3, label="Bar plot") p4 = histogram(y4, label="Histogram") plot(p1, p2, p3, p4, layout=(2, 2))
Interactive and High-Performance Visualization with Makie.jl
Makie.jl is a data visualization package that offers high-performance rendering and interactivity. To get started with Makie.jl, you first need to install the package:
import Pkg Pkg.add("Makie")
Now, you can use the lines
function to create a simple line plot:
using Makie x = 0:0.1:2π y = sin.(x) figure = lines(x, y, linewidth=2, color=:blue) # Save the plot as a PNG file save("sine_plot.png", figure)
Makie.jl supports various types of plots, including line plots, scatter plots, bar plots, histograms, and more. You can customize the appearance of the plots by specifying various attributes, such as colors, markers, and line styles.
For example, to create an interactive scatter plot with custom markers and colors, you can use the following code:
x = 1:10 y = rand(10) figure = scatter(x, y, markersize=10, markercolor=:red) # Save the plot as a PNG file save("scatter_plot.png", figure)
Creating Subplots with Makie.jl
You can create subplots using Makie.jl's grid
function, which arranges the plots in a grid layout. For example, to create a 2x2 grid of subplots with different types of plots, you can use the following code:
using Makie x = 1:10 y1 = rand(10) y2 = rand(10) y3 = rand(10) y4 = rand(10) p1 = lines(x, y1, color=:blue) p2 = scatter(x, y2, markercolor=:red) p3 = bar(x, y3, color=:green) p4 = histogram(y4, color=:purple) figure = grid(layout=GridLayout(2, 2), p1, p2, p3, p4) # Save the plot as a PNG file save("subplot_grid.png", figure)
Conclusion
In this post, we introduced data visualization in Julia using the Plots.jl and Makie.jl packages. We demonstrated how to create various types of plots, including line plots, scatter plots, bar plots, histograms, and subplots, with various customizations and interactivity options. With these tools, you can create insightful visualizations to analyze and present your data effectively.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from statistical analysis and time series forecasting to advanced numerical computing and scientific applications. Keep learning, and happy coding!