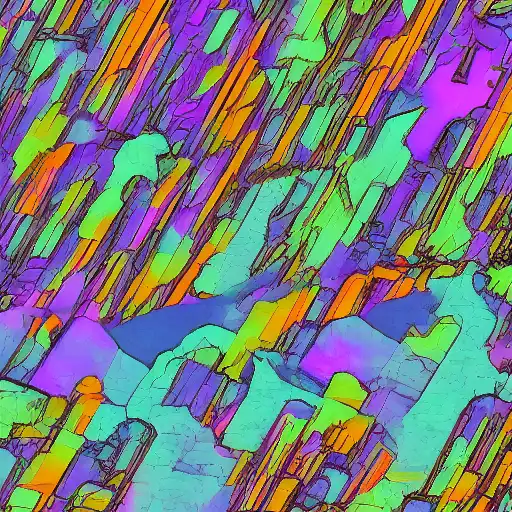
June 17th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, and building web applications. In this post, we will delve into web scraping in Julia, exploring how to fetch and parse web content using the HTTP.jl and Gumbo.jl packages.
Overview of Web Scraping Packages in Julia
Web scraping is the process of extracting data from websites by fetching web pages, parsing the HTML content, and extracting the desired information. In Julia, there are several packages available for web scraping, including:
- HTTP.jl: A package for making HTTP requests, which can be used to fetch web pages.
- Gumbo.jl: A package for parsing HTML content, based on the Google-developed Gumbo library.
- Cascadia.jl: A package for selecting HTML elements using CSS selectors, which can be used in conjunction with Gumbo.jl.
In this post, we will focus on using HTTP.jl for fetching web pages and Gumbo.jl for parsing the HTML content.
Fetching Web Pages with HTTP.jl
To fetch a web page using HTTP.jl, you first need to install the package:
import Pkg Pkg.add("HTTP")
Now, you can use the HTTP.get
function to fetch a web page:
using HTTP url = "https://example.com" response = HTTP.get(url) # Check if the request was successful if response.status == 200 println("Successfully fetched the web page!") else println("Failed to fetch the web page. Status code: ", response.status) end
The HTTP.get
function returns an HTTP.Response
object, which contains the HTTP status code, headers, and body (the HTML content of the web page). To access the HTML content, you can use the response.body
attribute:
html_content = String(response.body)
Parsing HTML Content with Gumbo.jl
To parse the HTML content of a web page, you can use the Gumbo.jl package. First, let's install the package:
import Pkg Pkg.add("Gumbo")
Now, you can use the Gumbo.parsehtml
function to parse the HTML content:
using Gumbo html_document = Gumbo.parsehtml(html_content)
The Gumbo.parsehtml
function returns an HTMLDocument
object, which represents the structure of the HTML content. The HTMLDocument
object has a root
attribute, which is the root element of the HTML content (usually the <html>
element).
To traverse the HTML content, you can use the attributes and functions provided by the Gumbo.jl package:
element.children
: A list of child elements of the given element.element.attributes
: A dictionary of attributes of the given element.Gumbo.bytag(element, tag_name)
: A function that returns a list of elements with the given tag name.
For example, to extract all the links from a web page, you can use the following code:
# Find all the <a> elements link_elements = Gumbo.bytag(html_document.root, "a") # Extract the href attribute of each <a> element links = [link.attributes["href"] for link in link_elements if haskey(link.attributes, "href")] # Print the links for link in links println(link) end
Using Cascadia.jl for Selecting HTML Elements
To select HTML elements using CSS selectors, you can use the Cascadia.jl package in conjunction with Gumbo.jl. First, let's install the package:
import Pkg Pkg.add("Cascadia")
Now, you can use the Cascadia.select
function to select elements based on a CSS selector:
using Cascadia # Define a CSS selector selector = "div.article > h2" # Select the elements that match the CSS selector selected_elements = Cascadia.select(html_document.root, selector) # Print the text content of the selected elements for element in selected_elements println(Gumbo.text(element)) end
The Cascadia.select
function returns a list of elements that match the given CSS selector. You can then extract the desired information from the selected elements using the attributes and functions provided by the Gumbo.jl package.
Conclusion
In this post, we introduced web scraping in Julia using the HTTP.jl and Gumbo.jl packages. We demonstrated how to fetch web pages, parse HTML content, and extract information from the parsed content using various techniques. With these tools, you can efficiently extract data from websites, making it easier to analyze and process the information as needed.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from data visualization and statistical analysis to advanced numerical computing and scientific applications. Keep learning, and happy coding!