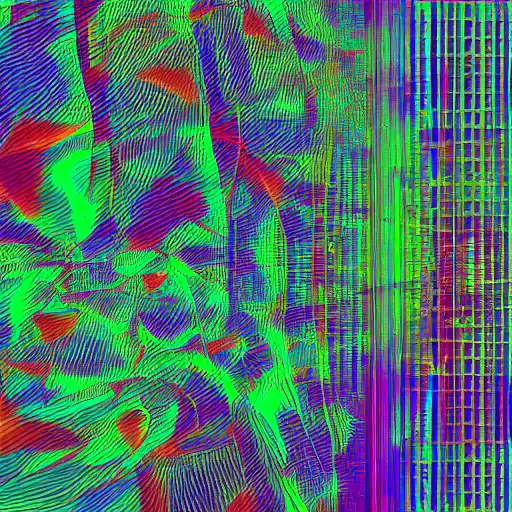
June 16th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, and working with databases. In this post, we will focus on building web applications in Julia, introducing you to the Genie web framework and HTTP.jl package.
Overview of Genie Web Framework
Genie is a full-stack web framework for Julia that enables you to build modern, high-performance web applications. With Genie, you can create web applications, RESTful APIs, and real-time web services with ease. It is inspired by Ruby on Rails and follows the Model-View-Controller (MVC) architectural pattern.
To get started with Genie, you first need to install the Genie package:
import Pkg Pkg.add("Genie")
Creating a Genie Application
To create a new Genie application, you can use the genie
command-line tool. First, you need to load the Genie package and then create a new application:
using Genie # Create a new Genie application Genie.newapp("MyWebApp")
This command will generate a new Genie application in the "MyWebApp" directory. Now, navigate to the application directory and start the Genie server:
cd MyWebApp julia --project -e 'using Genie; Genie.startup()'
By default, the Genie server will run on port 8000. You can access the web application by opening a browser and navigating to "http://localhost:8000".
Building a Simple Web Application with Genie
In this section, we will create a simple web application that allows users to view and add messages. First, let's create a new controller and a route for the messages:
using Genie.Router, Genie.Renderer.Json route("/messages", method = GET) do # Fetch messages from the database (not implemented yet) messages = get_messages() # Render the messages as JSON json(messages) end
Now, let's create a function to add a new message to the database:
route("/messages", method = POST) do # Get the message from the request body (not implemented yet) message = get_message_from_request() # Add the message to the database (not implemented yet) add_message(message) # Return a success message json("Message added successfully!") end
Next, we will create a simple user interface for our application. In the "views" directory, create a new file named "messages.html.erb":
<!DOCTYPE html> <html> <head> <title>Messages</title> </head> <body> <h1>Messages</h1> <form action="/messages" method="post"> <label for="message">Message:</label> <input type="text" id="message" name="message"> <button type="submit">Add Message</button> </form> <ul> <% for message in @messages %> <li><%= message %></li> <% end %> </ul> </body> </html>
Now, update the "messages" route in the controller to render the "messages.html.erb" view:
route("/messages", method = GET) do # Fetch messages from the database (not implemented yet) messages = get_messages() # Render the messages.html.erb view html(:messages, messages = messages) end
To complete the application, you'll need to implement the get_messages()
, get_message_from_request()
, and add_message()
functions, which interact with the database to store and retrieve messages. You can refer to our previous post on working with databases in Julia to learn how to do this.
Using HTTP.jl for Building Web Applications and APIs
Another way to build web applications and APIs in Julia is to use the HTTP.jl package, a high-level and low-level HTTP client and server implementation. To get started, you need to install the HTTP.jl package:
import Pkg Pkg.add("HTTP")
You can create a simple web server using HTTP.jl by defining a request handler function and starting the server:
using HTTP function request_handler(request) # Process the request and generate a response response = HTTP.Response("Hello, World!") # Return the response return response end # Start the HTTP server HTTP.serve(request_handler, "localhost", 8000)
To build a more sophisticated web application or API, you can use the HTTP.Router submodule, which provides a simple way to define routes and route handlers:
using HTTP, HTTP.Router # Create a new router router = Router() # Define a route handler function messages_handler(request) # Fetch messages from the database (not implemented yet) messages = get_messages() # Return the messages as JSON return HTTP.Response(200, JSON3.write(messages)) end # Add the route handler to the router route(router, "/messages", messages_handler) # Start the HTTP server HTTP.serve(router, "localhost", 8000)
As with the Genie example, you'll need to implement the get_messages()
function to interact with the database and fetch the messages.
Conclusion
In this post, we introduced the Genie web framework and HTTP.jl package for building web applications and APIs in Julia. With these tools, you can create modern, high-performance web applications, leveraging Julia's capabilities in scientific computing, data analysis, and machine learning.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from web scraping and data visualization to advanced numerical computing and scientific applications. Keep learning, and happy coding!