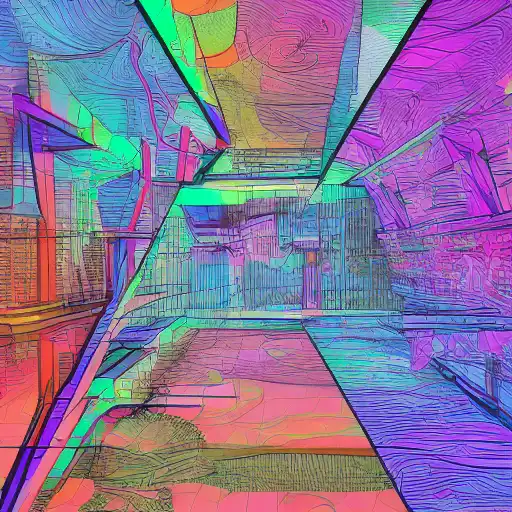
June 15th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. In this series, we have covered a variety of topics, including setting up a coding environment, syntax and unique features, data science, advanced machine learning techniques, and optimization strategies. In this post, we will focus on working with databases in Julia, exploring how to connect, query, and manage data efficiently.
Overview of Database Packages in Julia
Julia has a rich ecosystem of packages that make it easy to work with various database systems. Some popular database packages include:
- SQLite.jl: A package for working with SQLite databases.
- MySQL.jl: A package for working with MySQL and MariaDB databases.
- PostgreSQL.jl: A package for working with PostgreSQL databases.
- ODBC.jl: A package for working with databases using the ODBC interface, which can connect to many database systems such as SQL Server, Oracle, and more.
In this post, we will demonstrate working with SQLite, MySQL, and PostgreSQL databases using their respective Julia packages. For other databases, you may use the ODBC.jl package or search for a dedicated package if available.
SQLite Database with SQLite.jl
SQLite is a lightweight, serverless, self-contained SQL database engine. To work with SQLite databases in Julia, you can use the SQLite.jl package. First, let's install the package:
import Pkg Pkg.add("SQLite")
Now let's create a new SQLite database, establish a connection, and create a table:
using SQLite # Create a new SQLite database db = SQLite.DB("my_database.sqlite") # Create a table SQLite.execute(db, """ CREATE TABLE IF NOT EXISTS users ( id INTEGER PRIMARY KEY, name TEXT NOT NULL, age INTEGER ); """)
To insert data into the table, you can use the execute
function:
# Insert data into the table SQLite.execute(db, "INSERT INTO users (name, age) VALUES ('Alice', 30);")
To query data from the table, you can use the SQLite.Query
object:
# Query data from the table query = SQLite.Query(db, "SELECT * FROM users;") for row in query println("ID: ", row[:id], ", Name: ", row[:name], ", Age: ", row[:age]) end
When you are done working with the SQLite database, you can close the connection:
# Close the connection SQLite.close(db)
MySQL Database with MySQL.jl
MySQL is a widely used, open-source relational database management system. To work with MySQL databases in Julia, you can use the MySQL.jl package. First, let's install the package:
import Pkg Pkg.add("MySQL")
Now let's establish a connection to a MySQL database and create a table:
using MySQL # Connect to a MySQL database conn = MySQL.connect("localhost", "username", "password", db="my_database") # Create a table MySQL.execute!(conn, """ CREATE TABLE IF NOT EXISTS users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, age INT ); """)
To insert data into the table, you can use the execute!
function:
# Insert data into the table MySQL.execute!(conn, "INSERT INTO users (name, age) VALUES ('Alice', 30);")
To query data from the table, you can use the MySQL.Query
object:
# Query data from the table query = MySQL.Query(conn, "SELECT * FROM users;") for row in query println("ID: ", row[:id], ", Name: ", row[:name], ", Age: ", row[:age]) end
When you are done working with the MySQL database, you can close the connection:
# Close the connection MySQL.disconnect(conn)
PostgreSQL Database with PostgreSQL.jl
PostgreSQL is a powerful, open-source object-relational database system. To work with PostgreSQL databases in Julia, you can use the PostgreSQL.jl package. First, let's install the package:
import Pkg Pkg.add("PostgreSQL")
Now let's establish a connection to a PostgreSQL database and create a table:
using PostgreSQL # Connect to a PostgreSQL database conn = PostgreSQL.connect("dbname=my_database user=username password=password") # Create a table PostgreSQL.execute(conn, """ CREATE TABLE IF NOT EXISTS users ( id SERIAL PRIMARY KEY, name VARCHAR(255) NOT NULL, age INT ); """)
To insert data into the table, you can use the execute
function:
# Insert data into the table PostgreSQL.execute(conn, "INSERT INTO users (name, age) VALUES ('Alice', 30);")
To query data from the table, you can use the PostgreSQL.Query
object:
# Query data from the table query = PostgreSQL.Query(conn, "SELECT * FROM users;") for row in query println("ID: ", row[:id], ", Name: ", row[:name], ", Age: ", row[:age]) end
When you are done working with the PostgreSQL database, you can close the connection:
# Close the connection PostgreSQL.disconnect(conn)
Conclusion
In this post, we explored working with databases in Julia, demonstrating how to connect, query, and manage data in SQLite, MySQL, and PostgreSQL databases using their respective Julia packages. These techniques will enable you to efficiently manage and analyze data in various database systems, expanding your capabilities as a Julia developer.
As we continue our series on Julia, stay tuned for more posts covering a wide range of topics, from web development and API integration to advanced numerical computing and scientific applications. Keep learning, and happy coding!