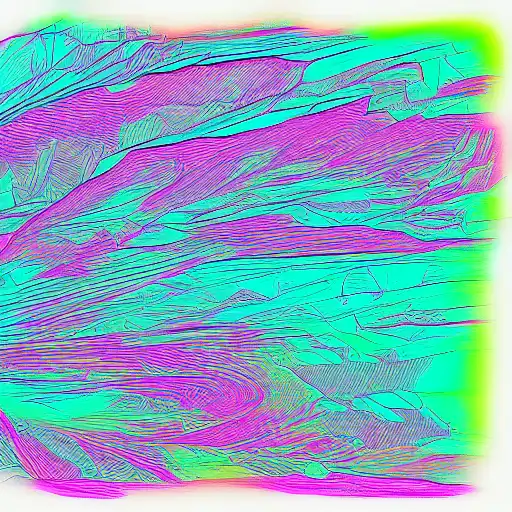
June 14th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. In this series, we've covered setting up a coding environment, discussed Julia's syntax and unique features, explored data science and advanced machine learning topics, and delved into parallel and distributed computing. In this post, we'll focus on advanced optimization techniques in Julia, which will help you write more efficient and high-performance code.
Profiling Your Julia Code
Before optimizing your code, it's important to identify the bottlenecks that are slowing it down. Profiling is a technique used to measure the performance of your code and find areas that need improvement. In Julia, you can use the built-in @profile
macro to profile your code:
using Profile function my_function(x) sleep(0.5) return x^2 end function main() for i in 1:10 my_function(i) end end # Profile the main function @profile main() # Display the profiling results Profile.print()
This example demonstrates how to profile your code using the @profile
macro and display the results using the Profile.print()
function. The output will show you which functions are consuming the most time, helping you to identify areas that need optimization.
Type Stability
One of the key factors that can impact the performance of your Julia code is type stability. Type stability refers to the property of a function that ensures its output type can be determined from its input types. Functions with unstable types can slow down your code, as Julia's just-in-time (JIT) compiler cannot optimize them effectively.
To check the type stability of your functions, you can use the @code_warntype
macro:
function unstable_function(x) if x > 0 return x^2 else return "Negative number" end end @code_warntype unstable_function(1)
In this example, the unstable_function
has an unstable output type, as it can return either an integer or a string. The @code_warntype
macro shows a warning, indicating that the output type cannot be inferred from the input type.
To optimize your code for performance, you should strive to write type-stable functions.
SIMD and Loop Vectorization
Single Instruction Multiple Data (SIMD) is a technique that enables a processor to perform the same operation on multiple data points simultaneously. Julia can automatically vectorize your loops, taking advantage of SIMD for improved performance.
To ensure that your loops can be vectorized, you should avoid using complex control structures or functions with side effects. Additionally, you can use the @simd
macro to explicitly indicate that a loop can be vectorized:
using Base.Threads function simd_example(data) result = zeros(length(data)) @threads for i in 1:length(data) @simd for j in 1:length(data) result[i] += data[j] * sin(j) end end return result end data = rand(1000) result = simd_example(data)
In this example, we use the @simd
macro to indicate that the inner loop can be vectorized. This allows Julia to take advantage of SIMD instructions for improved performance.
Efficient Memory Allocation
Memory allocation can have a significant impact on the performance of your Julia code. By minimizing memory allocations and reusing memory, you can greatly improve the efficiency of your code.
One way to reduce memory allocations is to use in-place operations whenever possible. For example, instead of creating a new array by adding two existing arrays, you can update one of the arrays in-place:
function in_place_example!(A, B) A .+= B end A = rand(1000) B = rand(1000) # Update A in-place by adding B in_place_example!(A, B)
In this example, we use the .+=
operator to perform an element-wise addition of A
and B
in-place, updating the elements of A
without creating a new array.
Another way to reduce memory allocations is to preallocate memory for temporary variables. If you have a loop that creates temporary arrays, you can preallocate memory for these arrays and reuse it in each iteration:
function preallocate_example(data, output) temp = similar(data) for i in 1:length(data) temp .= data .^ i output[i] = sum(temp) end end data = rand(1000) output = zeros(length(data)) # Use preallocated memory for the temporary variable preallocate_example(data, output)
In this example, we preallocate memory for the temp
array and reuse it in each iteration of the loop. This reduces memory allocations and improves the performance of the code.
Conclusion
In this post, we've explored advanced optimization techniques in Julia that can help you write more efficient and high-performance code. By profiling your code, ensuring type stability, taking advantage of SIMD and loop vectorization, and minimizing memory allocations, you can greatly improve the performance of your Julia programs.
Throughout this series, we've covered a wide range of topics in Julia, from setting up a coding environment to parallel and distributed computing, data science, and advanced machine learning techniques. However, there is still much more to learn and explore in the world of Julia. In the remaining posts of this series, we will delve further into various aspects of the language and its ecosystem, helping you to become a more proficient and well-rounded Julia developer.
Stay tuned for the upcoming posts as we continue our journey through Julia's powerful features and capabilities. Keep learning, and happy coding!