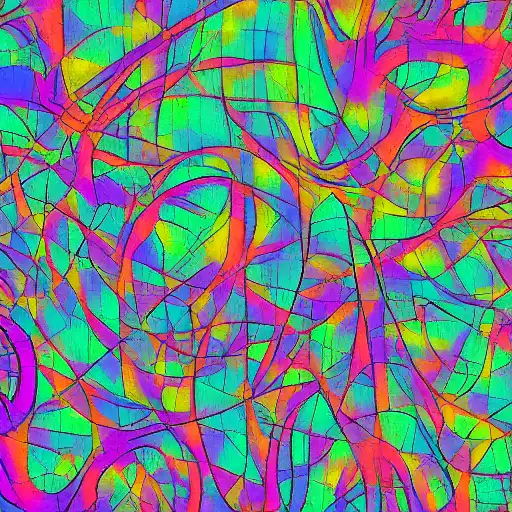
June 13th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. Throughout this series, we've covered setting up a coding environment, discussed Julia's syntax and unique features, and explored using Julia for data science and advanced machine learning tasks. In this post, we'll dive into parallel and distributed computing with Julia, demonstrating how you can take advantage of multicore processors and distributed computing environments to speed up your computations.
Parallel Computing with Julia
Julia has built-in support for parallel computing, allowing you to easily take advantage of multicore processors. In this section, we'll cover two main parallel programming constructs in Julia: @spawn
and @threads
.
Using @spawn
for Asynchronous Tasks
@spawn
is a macro that allows you to asynchronously execute a task on any available processor. To use @spawn, you'll first need to add worker processes:
using Distributed addprocs(4) # Add 4 worker processes
Next, you can use the @spawn
macro to create a task that runs asynchronously:
function slow_function(x) sleep(2) return x^2 end # Spawn a task to run the slow_function async_task = @spawn slow_function(10) # Fetch the result when it's ready result = fetch(async_task) println("Result: $result")
In this example, the slow_function
is executed asynchronously on a worker process. The fetch
function waits for the task to complete and retrieves the result.
Using @threads
for Multi-threading
@threads
is a macro that enables you to parallelize a loop using multiple threads. To use @threads
, you'll first need to set the number of threads in your environment:
export JULIA_NUM_THREADS=4
Then, you can use the @threads
macro to parallelize a loop:
using Base.Threads data = collect(1:10) squared_data = zeros(Int, length(data)) @threads for i in 1:length(data) squared_data[i] = data[i]^2 end println("Squared data: $squared_data")
In this example, the loop is parallelized using multiple threads, and each iteration is executed by an available thread.
Distributed Computing with Julia
In addition to parallel computing, Julia supports distributed computing, allowing you to distribute your computations across multiple computers in a cluster. In this section, we'll discuss two main concepts in distributed computing with Julia: remote references and remote channels.
Remote References
Remote references are used to store the results of computations on remote processes. You can create a remote reference using the remotecall
function:
using Distributed function slow_function(x) sleep(2) return x^2 end # Call the slow_function on worker 2 remote_result = remotecall(slow_function, 2, 10) # Fetch the result result = fetch(remote_result) println("Result: $result")
In this example, the slow_function
is executed on worker 2, and the result is stored in a remote reference. The fetch
function is used to retrieve the result.
Remote Channels
Remote channels provide a way to communicate and synchronize tasks between different processes. You can create a remote channel and use it to send and receive data between processes:
using Distributed # Create a remote channel channel = RemoteChannel(()->Channel{Int}(10)) # Define a function that sends data to the remote channel function send_data(channel, data) for item in data put!(channel, item) end close(channel) end # Define a function that receives data from the remote channel and squares it function receive_and_square_data(channel) squared_data = Int[] for item in channel push!(squared_data, item^2) end return squared_data end data = collect(1:10) # Spawn a task to send data to the remote channel @spawn send_data(channel, data) # Spawn a task to receive and square data from the remote channel squared_data_task = @spawn receive_and_square_data(channel) # Fetch the squared data squared_data = fetch(squared_data_task) println("Squared data: $squared_data")
In this example, we create a remote channel and use it to send and receive data between two tasks running on different processes. The data is squared by the receive_and_square_data
function, and the results are retrieved using the fetch
function.
Conclusion
In this post, we explored parallel and distributed computing with Julia, demonstrating how to take advantage of multicore processors and distributed computing environments to speed up your computations. With these tools, you can improve the performance of your Julia code and tackle large-scale problems more efficiently.
Throughout this series, we've covered a wide range of topics in Julia, from setting up a coding environment to advanced machine learning techniques and parallel processing. We hope this series has provided you with a solid foundation to continue exploring Julia and utilizing its powerful features in your projects. Keep learning, and happy coding!