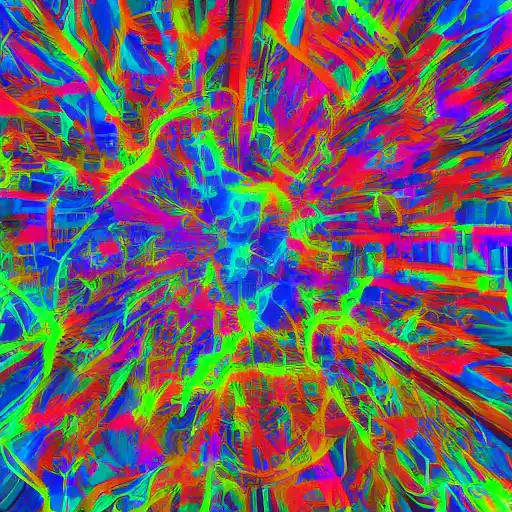
June 12th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. So far, we've covered setting up a coding environment, discussed Julia's syntax and unique features, and explored using Julia for data science tasks. In this post, we'll dive into advanced machine learning topics, focusing on deep learning with Flux.jl and natural language processing (NLP) with TextAnalysis.jl.
Deep Learning with Flux.jl
Flux.jl is a powerful and user-friendly package for deep learning in Julia. It features lightweight syntax, GPU support, and a flexible design. To get started, install Flux.jl and its dependencies using the following command:
using Pkg Pkg.add("Flux")
Next, import the package by running:
using Flux
Building a Simple Neural Network
With Flux.jl, you can easily define, train, and evaluate neural networks. Let's create a simple feedforward neural network for classifying handwritten digits using the famous MNIST dataset:
using Flux, Flux.Data.MNIST # Load the data images = Flux.Data.MNIST.images() labels = Flux.Data.MNIST.labels() # Preprocess the data X = hcat(float.(reshape.(images, :))...) |> gpu Y = onehotbatch(labels, 0:9) |> gpu # Split the data into training and validation sets train_indices = 1:50_000 val_indices = 50_001:length(labels) X_train = X[:, train_indices] Y_train = Y[:, train_indices] X_val = X[:, val_indices] Y_val = Y[:, val_indices] # Define the neural network model = Chain( Dense(28^2, 128, relu), Dense(128, 64, relu), Dense(64, 10), softmax ) |> gpu # Define the loss function loss(x, y) = Flux.Losses.logitcrossentropy(model(x), y) # Define the optimizer opt = ADAM() # Train the model dataset = Flux.Data.DataLoader((X_train, Y_train), batchsize=128, shuffle=true) Flux.train!(loss, Flux.params(model), dataset, opt) # Evaluate the model accuracy(x, y) = mean(onecold(cpu(model(x))) .== onecold(cpu(y))) println("Validation accuracy: $(accuracy(X_val, Y_val))")
This example demonstrates how to load and preprocess the MNIST dataset, define a simple feedforward neural network, train the network using the ADAM optimizer, and evaluate the model on a validation set.
Natural Language Processing with TextAnalysis.jl
TextAnalysis.jl is a comprehensive package for NLP tasks in Julia. It offers tools for text preprocessing, feature extraction, and text classification. To get started, install TextAnalysis.jl using the following command:
using Pkg Pkg.add("TextAnalysis")
Next, import the package:
using TextAnalysis
Text Preprocessing and Feature Extraction
Let's preprocess a corpus of text documents and extract features using TextAnalysis.jl:
using TextAnalysis # Create a corpus of documents docs = ["This is the first document.", "This is the second document.", "And this is the third one.", "Is this the first document?"] corpus = Corpus(StringDocument.(docs)) # Preprocess the text prepare!(corpus, strip_whitespace | strip_punctuation | strip_case) # Create a term-document matrix term_doc_matrix = DocumentTermMatrix(corpus) # Convert the term-document matrix to a matrix of term frequencies tf_matrix = tf(term_doc_matrix, normalize=true) # Apply the inverse document frequency (IDF) transformation idf_transformer = fit!(IDF(), tf_matrix) tfidf_matrix = transform(idf_transformer, tf_matrix) # Convert the matrix to a dense format dense_tfidf_matrix = Matrix(tfidf_matrix)
This example demonstrates how to create a corpus of documents, preprocess the text, create a term-document matrix, compute term frequencies, and apply the inverse document frequency (IDF) transformation. The resulting matrix contains the TF-IDF features for each document.
Text Classification
using TextAnalysis, Random # Prepare the training data labels = ["A", "A", "B", "A"] data = [(docs[i], labels[i]) for i in 1:length(labels)] # Shuffle the data Random.seed!(1234) shuffle!(data) # Split the data into training and validation sets train_data, val_data = data[1:3], data[4:end] # Create the text classifier classifier = NaiveBayesClassifier() # Train the classifier fit!(classifier, train_data) # Evaluate the classifier correct = 0 for (doc, label) in val_data prediction = classify(classifier, doc) if prediction == label correct += 1 end end accuracy = correct / length(val_data) println("Validation accuracy: $accuracy")
This example shows how to prepare labeled text data, train a Naive Bayes classifier using TextAnalysis.jl, and evaluate the classifier on a validation set.
Conclusion
In this post, we explored advanced machine learning topics in Julia, including deep learning with Flux.jl and natural language processing with TextAnalysis.jl. These packages provide powerful tools for tackling complex machine learning problems and help you unlock the full potential of Julia in your projects.
With the knowledge you've gained from this series, you should be well-equipped to explore Julia's rich ecosystem and use it to solve a wide range of problems in scientific computing, data science, and machine learning. Keep learning, and happy coding!