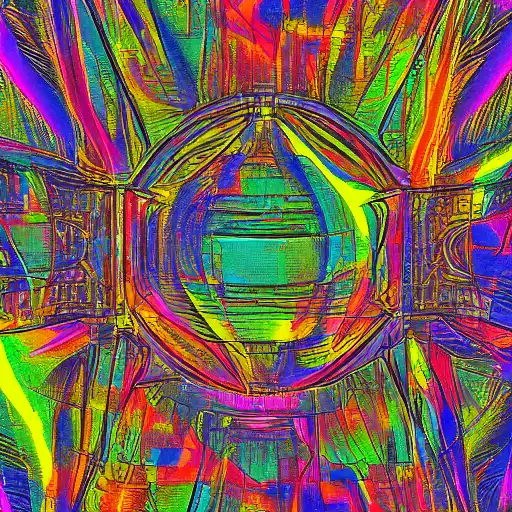
June 29th, 2023
Welcome back to our series on Julia, the high-performance programming language designed for scientific computing. We have covered various aspects of the language, including setting up a coding environment, syntax and unique features, data science, machine learning techniques, optimization strategies, working with databases, building web applications, web scraping, data visualization, time series forecasting, deep learning, mathematical optimization, scientific applications, advanced numerical computing, optimization and root-finding with NLsolve.jl, statistical modeling with GLM.jl, numerical integration with QuadGK.jl, machine learning with Flux.jl, data manipulation with DataFrames.jl, and optimization with JuMP.jl. In this final post of the series, we will focus on graph algorithms in Julia, introducing the LightGraphs.jl package and demonstrating various graph data structures, algorithms, and their applications in network analysis, transportation, social networks, and more.
Overview of Graph Packages in Julia
There are several graph packages available in Julia, including:
- LightGraphs.jl: A lightweight, high-performance, and easy-to-use package for working with graph data structures and algorithms in Julia.
- Graphs.jl: A more extensive package for graph processing, offering additional graph types, algorithms, and visualization capabilities, at the cost of increased complexity and lower performance compared to LightGraphs.jl.
- MetaGraphs.jl: An extension of LightGraphs.jl that adds metadata support to graph objects, allowing users to associate additional information with vertices and edges.
In this post, we will focus on LightGraphs.jl, which provides a comprehensive toolkit for data manipulation and transformation in Julia.
Getting Started with LightGraphs.jl
To get started with LightGraphs.jl, you first need to install the package:
import Pkg Pkg.add("LightGraphs")
Now, you can create a simple undirected graph:
using LightGraphs # Create an undirected graph with 5 vertices G = SimpleGraph(5) # Add edges to the graph add_edge!(G, 1, 2) add_edge!(G, 1, 3) add_edge!(G, 2, 3) add_edge!(G, 3, 4) add_edge!(G, 4, 5) # Display the graph println(G)
In this example, we create an undirected graph with five vertices and add edges between them using the add_edge!
function. The graph is displayed using the println
function, which shows the adjacency list representation of the graph.
Basic Graph Operations and Algorithms
LightGraphs.jl provides various functions for basic graph operations and algorithms:
using LightGraphs # Compute the degree of each vertex degrees = degree(G) # Check if the graph is connected is_connected = is_connected(G) # Compute the shortest path between two vertices shortest_path = shortest_path(G, 1, 5) # Compute the minimum spanning tree of the graph mst = kruskal_mst(G)
In this example, we demonstrate how to compute the degree of each vertex, check if the graph is connected, find the shortest path between two vertices, and compute the minimum spanning tree of the graph.
Advanced Graph Algorithms and Applications
LightGraphs.jl also provides functions for advanced graph algorithms and applications, such as centrality measures, clustering coefficients, community detection, and more:
using LightGraphs # Compute betweenness centrality betweenness_centrality = betweenness_centrality(G) # Compute closeness centrality closeness_centrality = closeness_centrality(G) # Compute the clustering coefficient of the graph clustering_coefficient = global_clustering_coefficient(G) # Perform community detection using the Louvain algorithm communities = louvain(G) # Compute the PageRank of each vertex pagerank_scores = pagerank(G) # Find the maximum flow in a directed graph flow_graph = SimpleDiGraph(5) add_edge!(flow_graph, 1, 2, 10) add_edge!(flow_graph, 1, 3, 5) add_edge!(flow_graph, 2, 3, 20) add_edge!(flow_graph, 2, 4, 5) add_edge!(flow_graph, 3, 5, 10) add_edge!(flow_graph, 4, 5, 20) max_flow = maximum_flow(flow_graph, 1, 5, capacity_matrix(flow_graph))
In this example, we demonstrate how to compute various centrality measures, such as betweenness and closeness centrality, clustering coefficients, community detection using the Louvain algorithm, PageRank scores, and maximum flow in a directed graph.
Conclusion
In this final post of our series on Julia, we introduced graph algorithms in Julia using the LightGraphs.jl package. We demonstrated various graph data structures, algorithms, and their applications in network analysis, transportation, social networks, and more. LightGraphs.jl provides a powerful and efficient framework for working with graph data structures and algorithms in Julia, enabling users to tackle complex problems in various domains.
Throughout the series, we have explored various aspects of the Julia programming language, including its syntax, unique features, and numerous applications in data science, machine learning, optimization, and other fields. We hope that this series has provided you with the knowledge and skills required to become a proficient Julia programmer and to tackle complex problems in your domain.
Thank you for joining us on this journey through the Julia programming language. Keep learning, and happy coding!