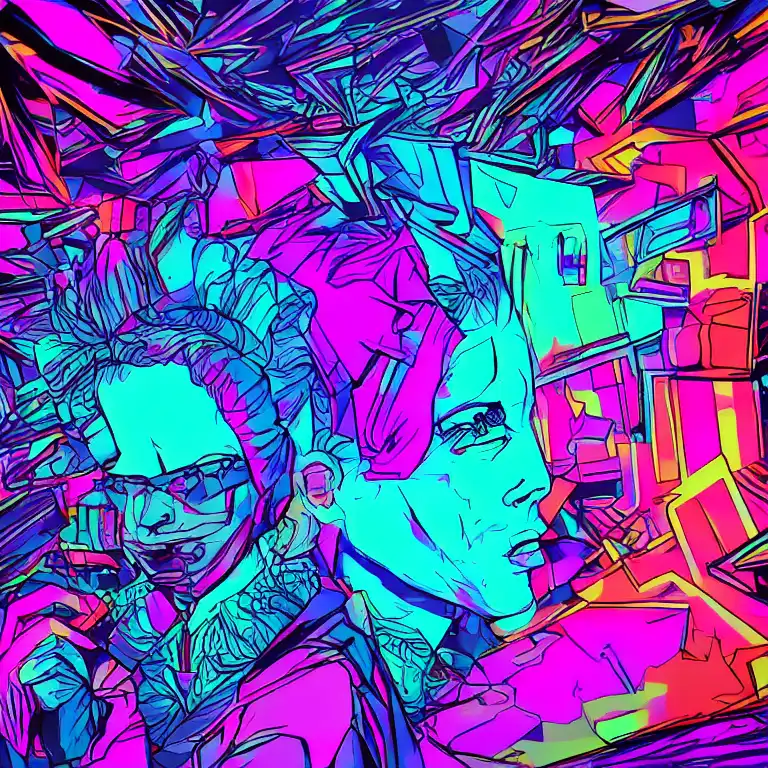
June 5th, 2023
Welcome back to our Go programming series! In our previous post, we covered the basics of using the Gin web framework to create a simple web application. Now, let's dive deeper into Gin and build a more complex web application.
Gin Routing
Gin provides a powerful and flexible routing system that allows you to handle incoming HTTP requests and route them to the appropriate handler functions. Here are a few examples of Gin routing:
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // create a new Gin router // handle GET requests to the root endpoint "/" router.GET("/", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{ "message": "Hello, World!", }) }) // handle GET requests to the "/users" endpoint router.GET("/users", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{ "users": []string{"Alice", "Bob", "Charlie"}, }) }) // handle GET requests to the "/users/:id" endpoint router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.JSON(http.StatusOK, gin.H{ "user": id, }) }) router.Run(":8080") // start the server on port 8080 }
In this example, we define three endpoint handlers using the router.GET
method.
The first handler handles GET requests to the root endpoint /
and returns a JSON response with the message "Hello, World!".
The second handler handles GET requests to the /users
endpoint and returns a JSON response with a list of users.
The third handler handles GET requests to the /users/:id
endpoint, where :id
is a dynamic parameter that can be accessed using the c.Param
method. This handler returns a JSON response with the user ID.
Gin Middleware
Gin middleware allows you to modify incoming HTTP requests and outgoing HTTP responses before they reach the endpoint handlers. Here's an example of using Gin middleware to add a custom header to every outgoing HTTP response:
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // create a new Gin router // add a custom header to every outgoing HTTP response router.Use(func(c *gin.Context) { c.Writer.Header().Set("X-App-Version", "1.0.0") c.Next() }) // handle GET requests to the root endpoint "/" router.GET("/", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{ "message": "Hello, World!", }) }) router.Run(":8080") // start the server on port 8080 }
In this example, we use the router.Use
method to add a middleware function that sets a custom header X-App-Version
to every outgoing HTTP response. We use the c.Writer.Header().Set
method to set the header value.
Conclusion
In this post, we covered Gin routing and middleware, two powerful features that allow you to build complex web applications with ease. In the next post, we'll dive into databases and show you how to connect your Go web application to a PostgreSQL database using the popular pgx
library. Stay tuned!