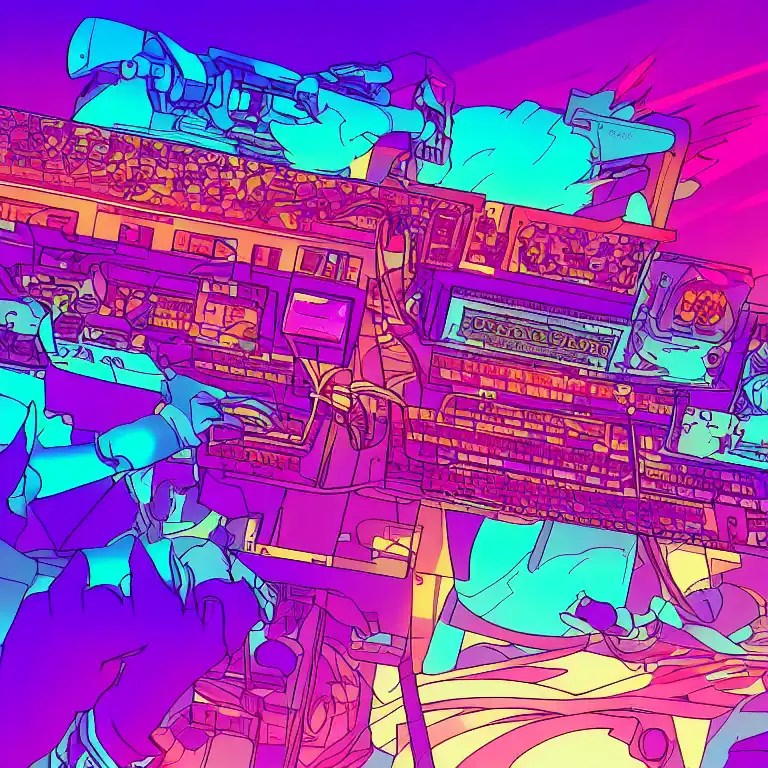
June 4th, 2023
Welcome back to our Go programming series! In our previous post, we covered Go's concurrency features, including goroutines and channels. Now, let's dive into the Gin web framework and build a basic web application.
Gin
Gin is a popular web framework for Go that provides a fast and easy way to build web applications. Here's an example of using Gin to create a basic web application:
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // create a new Gin router router.GET("/", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{ "message": "Hello, World!", }) }) router.Run(":8080") // start the server on port 8080 }
In this example, we import the Gin framework using the github.com/gin-gonic/gin
package.
We create a new Gin router using the gin.Default()
function. The router handles incoming HTTP requests and routes them to the appropriate handler functions.
We define a handler function for the root endpoint /
using the router.GET
method. This function returns a JSON response with the message "Hello, World!".
We start the server on port 8080 using the router.Run
method.
Now, let's run our web application. Open up a terminal, navigate to your workspace directory, and run the following commands:
cd $HOME/go/src go run main.go
This will start the server on port 8080. Open up your web browser and navigate to http://localhost:8080. You should see a JSON response with the message "Hello, World!".
Conclusion
In this post, we covered the basics of using the Gin web framework to create a simple web application. In the next post, we'll dive deeper into Gin and build a more complex web application. Stay tuned!