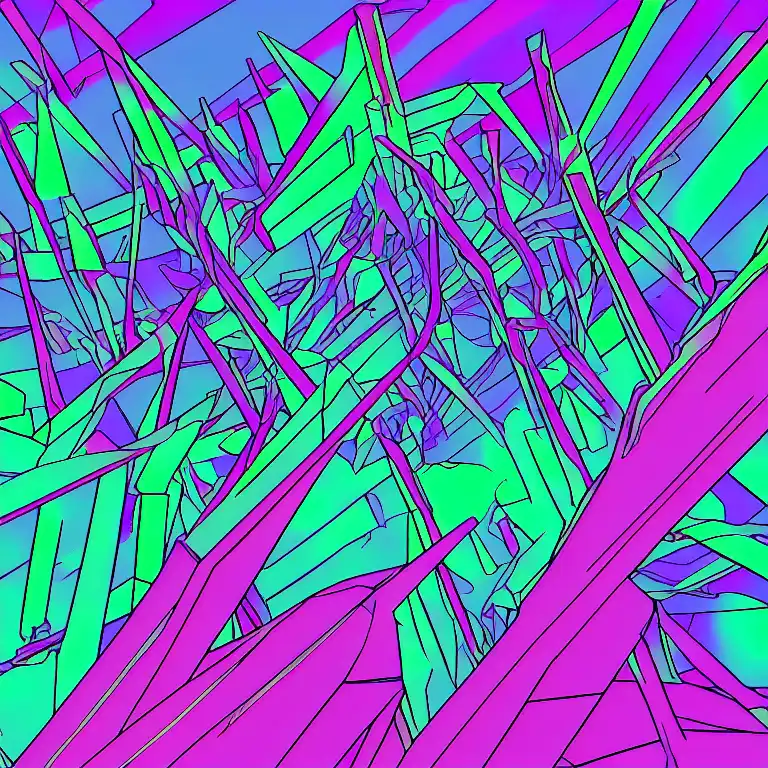
June 3rd, 2023
Welcome back to our Go programming series! In our previous post, we covered Go's control structures and functions. Now, let's dive into Go's concurrency features, which are one of its unique strengths.
Concurrency
Go has built-in support for concurrency, which means that you can easily write concurrent code to execute multiple tasks simultaneously. Here are a few examples of Go's concurrency features:
Goroutines
Goroutines are lightweight threads of execution that can run concurrently with other goroutines. Here's an example of defining and running a goroutine:
package main import ( "fmt" "time" ) func sayHello() { fmt.Println("Hello, World!") } func main() { go sayHello() // start a new goroutine time.Sleep(time.Second) // wait for the goroutine to finish }
In this example, we define a function sayHello
that prints "Hello, World!" to the console. We use the go
keyword to start a new goroutine running the sayHello
function.
We use the time.Sleep
function to wait for the goroutine to finish before the main
function exits. If we didn't wait for the goroutine to finish, the program would exit immediately without printing anything to the console.
Channels
Channels are a powerful tool for synchronizing access to shared data between goroutines. Here's an example of using a channel to send and receive data between goroutines:
package main import ( "fmt" "time" ) func sendMessage(c chan string) { c <- "Hello, World!" // send a message to the channel } func main() { c := make(chan string) // create a new channel go sendMessage(c) // start a new goroutine sending a message to the channel msg := <-c // receive the message from the channel fmt.Println(msg) }
In this example, we define a function sendMessage
that sends a message "Hello, World!" to a channel of type string
.
We create a new channel c
using the make function. We use the go
keyword to start a new goroutine running the sendMessage
function, sending a message to the channel.
We use the <-
operator to receive the message from the channel and store it in the msg
variable. We print the message to the console.
Conclusion
In this post, we covered Go's concurrency features, including goroutines and channels. In the next post, we'll dive into the Gin web framework and build a basic web application. Stay tuned!