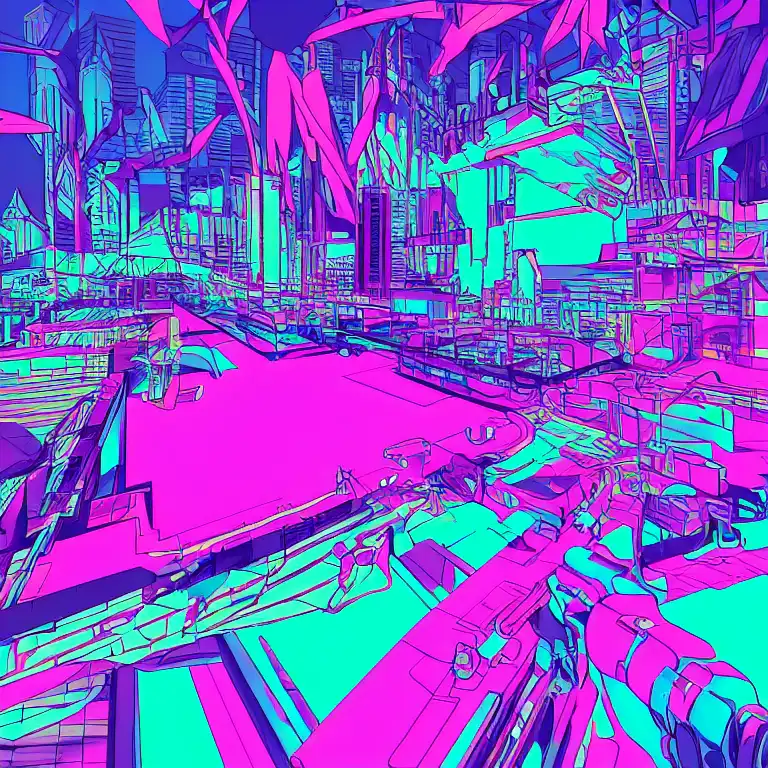
June 2nd, 2023
Welcome back to our Go programming series! In our previous post, we covered the basics of Go syntax and some of its unique features, including variables and types, and structs. Now, let's dive deeper into Go's control structures and functions.
Control Structures
Go has several control structures that you can use to control the flow of your code. Here are a few examples:
- If Statements
package main import "fmt" func main() { x := 10 if x < 5 { fmt.Println("x is less than 5") } else if x < 15 { fmt.Println("x is between 5 and 15") } else { fmt.Println("x is greater than or equal to 15") } }
In this example, we use an if
statement to check whether the value of x
is less than 5. If it is, we print "x is less than 5". If not, we check whether it's less than 15. If it is, we print "x is between 5 and 15". If not, we print "x is greater than or equal to 15".
- For Loops
package main import "fmt" func main() { for i := 0; i < 5; i++ { fmt.Println(i) } }
In this example, we use a for
loop to print the numbers from 0 to 4 to the console.
- Functions
Functions are an essential part of Go programming, and they allow you to encapsulate logic into reusable blocks of code. Here's an example of defining and using a function:
package main import "fmt" func add(x int, y int) int { return x + y } func main() { result := add(10, 5) fmt.Println(result) }
In this example, we define a function add
that takes two int
arguments and returns their sum.
We call the add
function in our main
function, passing in the values 10
and 5
. The function returns their sum, which we store in the result
variable.
Conclusion
In this post, we covered Go's control structures and functions. In the next post, we'll dive into Go's concurrency features, which are one of its unique strengths. Stay tuned!