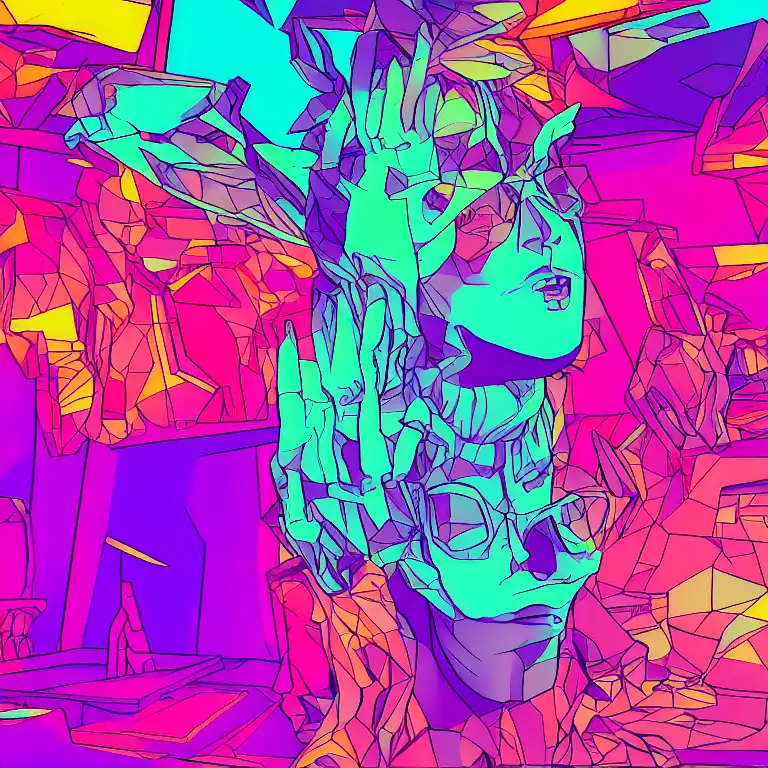
June 1st, 2023
Welcome back to our Go programming series! In our previous post, we set up our coding environment on Linux and created a Go workspace. Now that we're all set up, let's dive into the basics of Go syntax and some of its unique features.
Hello, World!
Let's start by creating our first Go program, the traditional "Hello, World!" program. Open up your favorite text editor and create a new file called hello.go
in your workspace directory ($HOME/go/src)
:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
The first line package main
tells Go that this is a main package, and the entry point of the program will be the main()
function.
Next, we import the fmt
package, which provides functions for formatting text.
In our main()
function, we call the Println()
function from the fmt
package to print the string "Hello, World!" to the console.
Now, let's compile and run our program. Open up a terminal, navigate to your workspace directory, and run the following commands:
cd $HOME/go/src go build hello.go ./hello
The go build
command compiles our Go code into an executable binary file, which we can run using the ./hello
command.
Variables && Types
Go is a statically typed language, which means that variables must be declared with their type at compile time. Here's an example of declaring and initializing variables in Go:
package main import "fmt" func main() { var a int = 5 var b float64 = 3.14 var c string = "Hello, World!" fmt.Printf("a = %d\n", a) fmt.Printf("b = %f\n", b) fmt.Printf("c = %s\n", c) }
In this example, we declare three variables a
, b
, and c
with their types int
, float64
, and string
, respectively. We initialize each variable with a value.
We use the fmt.Printf()
function to print the value of each variable. The %d
, %f
, and %s
format specifiers tell Go to print the values in decimal, float, and string formats, respectively.
Structs
In Go, structs are used to define custom data types. Here's an example of defining and using a struct:
package main import "fmt" type person struct { name string age int } func main() { p := person{name: "John", age: 30} fmt.Printf("Name: %s, Age: %d\n", p.name, p.age) }
In this example, we define a person
struct with two fields: name
of type string
and age
of type int
.
We create a new person
struct instance p
and initialize its name
and age
fields using the syntax p := person{name: "John", age: 30}
.
We use the fmt.Printf()
function to print the name
and age
fields of the p
struct instance.
Conclusion
In this post, we covered the basics of Go syntax and some of its unique features, including variables and types, and structs. In the next post, we'll dive deeper into Go's control structures and functions. Stay tuned!